How do you build static websites with Gatsby and (r) (r)
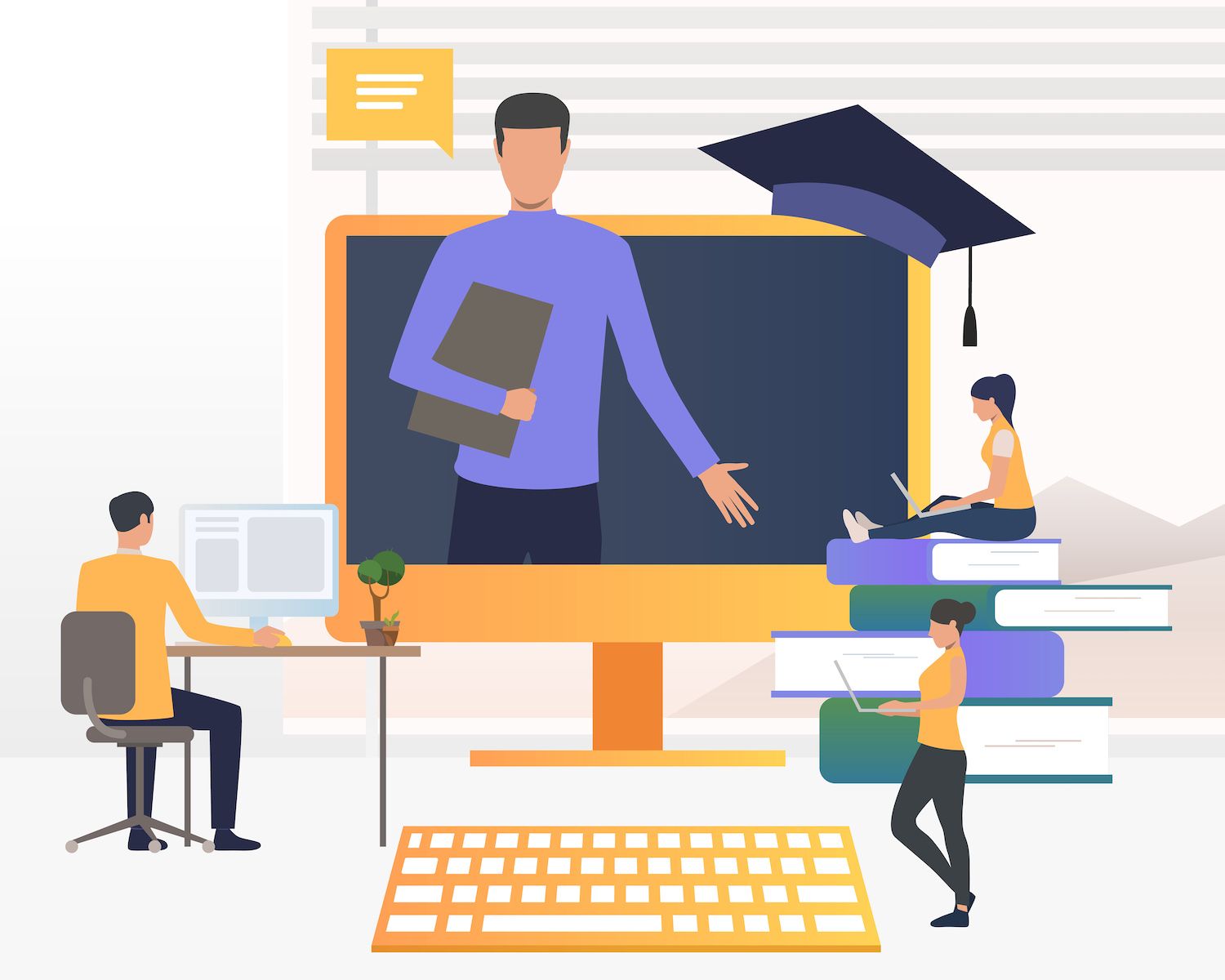
-sidebar-toc>
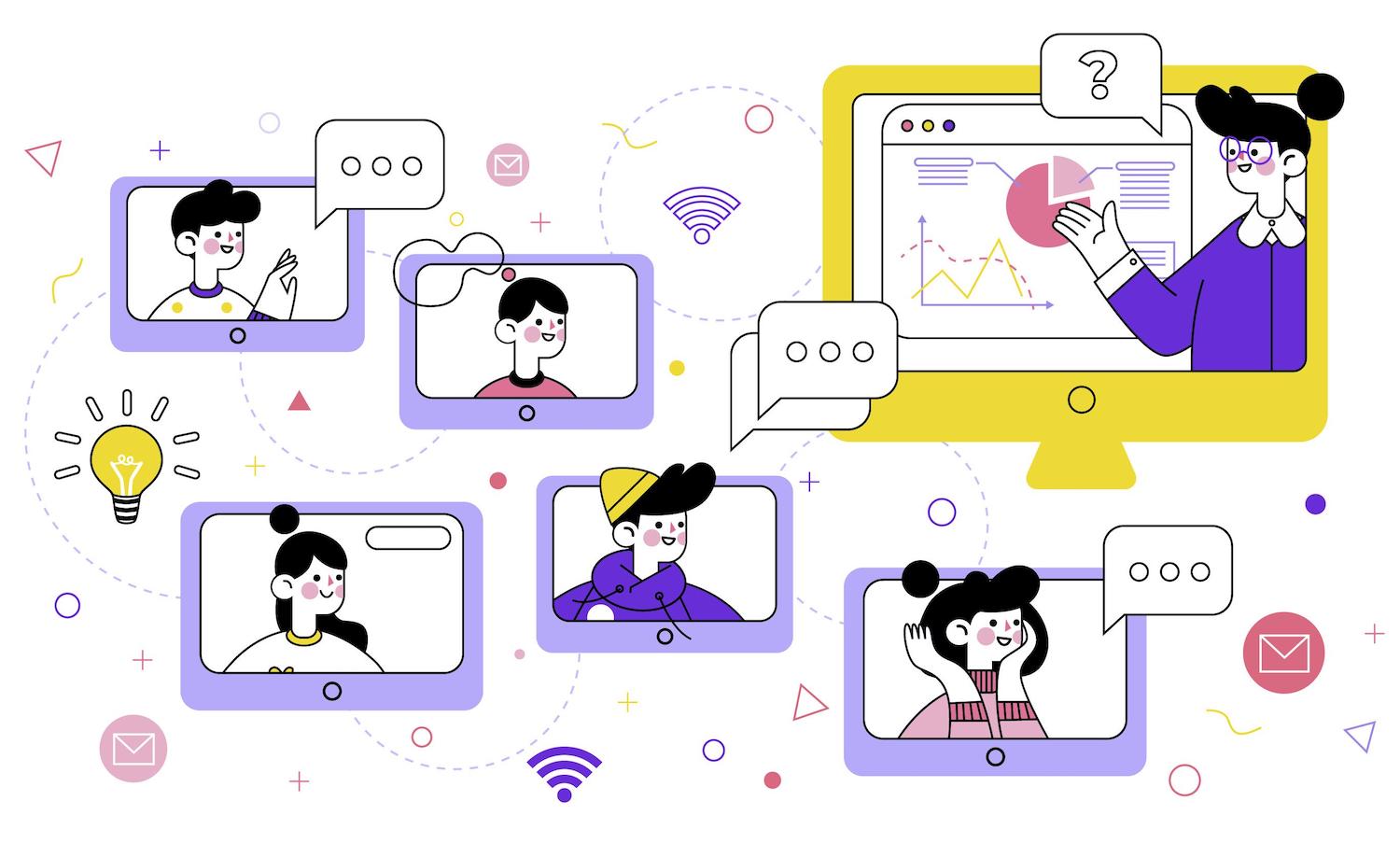
Understanding Static Sites
- Speed: Static sites load quickly since there's no server-side processing.
- Security As there is no executable code on the server, static sites are less susceptible to threats related to security.
- Scalability It's simple to share and cache static web pages via Content Delivery Networks (CDNs).
- Simplicity: They are easier to create, use and manage.
After you have a better understanding of what static sites are and their benefits let's dive into Gatsby.
What is Gatsby?
- Rapidly Fast: Gatsby optimizes your site for speed using techniques such as code splitting and lazy loading. This results in fast loading of your pages.
- Flexible data sourcing: It can get data from multiple sources such as Markdown files, APIs, databases and more.
- Rich Plug-in Ecosystem: Gatsby's large ecosystem of plugins allows you to extend the functionality of your application easily.
- SEO and performance: Gatsby automatically produces optimized HTML that is better suited to SEO and to perform.
Beginning to Get Started with Gatsby
For you to be able to comply to this instruction You must have:
- Basic understanding in HTML, CSS, and JavaScript
- Fundamental knowledge of React
If you want to start with Gatsby and develop a plan take a look at one of the hundreds of examples available in Gatsby Starter Library. Gatsby Starter Library as well as create an project from the beginning until completion.
To follow this procedure Let's make use of GatsbyJS's Hello World starter for GatsbyJS since it offers us an uncomplicated project that does not have extensions or other files.
- To begin, you must install Gatsby's CLI onto your PC using the below instruction:
NPM install -g gatsby cli
Run the gatsby version
to check if the installation was successful.
- Next, navigate into the directory you want to build your project in, and run the following command:
npx gatsby new https://github.com/gatsbyjs/gatsby-starter-hello-world
Change the project-name>
above to match the title for the project you're working on.
- After this has been completed After that, go to the folder for your project and begin the development server.
Cd gatsby create
The local development server will start at http://localhost:8000, where you can access your Gatsby site.
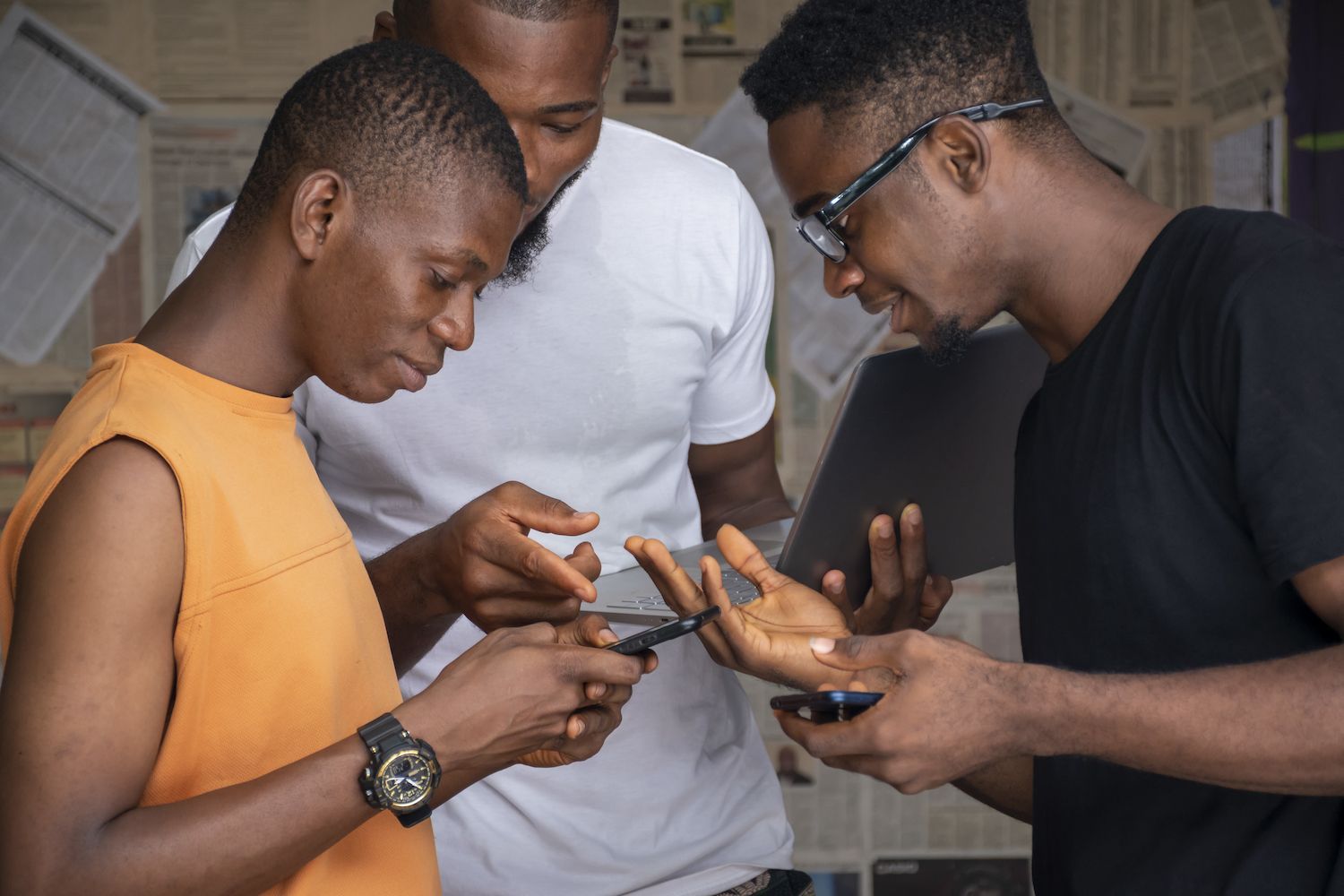
Learning Gatsby File Structure
When you open your project using an editor for code and you'll be able observe the following structure:
/ |-- /public |-- /src |-- /pages |-- index.js |-- /static |-- gatsby-config.js
- public: This directory contains the output of your Gatsby process of building. This is where your created HTML, CSS, JavaScript along with other data files are saved.
- (or src): This is the center of the Gatsby project. That's in which you'll have to spend much of the duration of your time. There are many subdirectories, as well as files:
- /pages: This is where every page for your website are stored. Every JavaScript file in this folder corresponds to the path you've set on your site.
- static This folder is utilized for static files that are needed to be added to your site, such as images, fonts or downloadable documents. They are provided as is and not processed by Gatsby.
- gatsby-config.js: This configuration file lets you define various settings for your Gatsby site. You can specify plugins or metadata for your site along with different settings.
Making Pages and Components
In Gatsby, building web pages is an easy process. Each JavaScript files you make in the pages/src directory is automatically transformed into a real page with the appropriate route, thanks to Gatsby's automated pages creation.
It is possible to create the pages you require on your site through the addition of additional JavaScript documents to the pages folder within the /src/pages folder. For instance, you can, build the about.js file for the "About" page.
Even though you're able make distinct JavaScript files for each page directly within the pages/src/pages folder, you are able to organize your pages in a different way. You can create subfolders to distinct pages. In this case, for example, you could create the blog folder that will organize every blog-related page.
For this project, this is the way that the page structure will look as follows:
|-- /src |-- /pages |-- about.js |-- index.js |-- /blog |-- index.js
Utilizing JSX for Pages
For example, you can create the content for your home Page ( index.js) by following the steps:
import React from'react";the default export method is Home() returning ( Enjoy Static Site Hosting With StSH. Quick, Secure and Reliable Hosting Solution. > );
Links Pages inside Gatsby
For creating a link to another website, you can utilize the Link
feature. the manner is:
import React into react; import Link from the 'gatsby' default function export default function for home() return ( Get Static Website Hosting with StSH. Rapid, secure, and reliable Hosting Solution. to="/about">About Us to="/blog">Blog > );
In this example in the above illustration, we've imported Link
elements from the gatsby website
and used it to build links for Our "About Us" page and blog. The "About Us" page, as an instance, we have the link about
. After users click on"About Us," like the "About Us" button and are taken to the page/about
page.
For creating links to external sites, use ordinary anchor ( ) tags with an the HREF
attribute:
import React using'react";Export the default home functions() return ( Enjoy Static Site Hosting With StSH. Rapid, secure, and reliable Hosting Solution. Take advantage of static site hosting with StSH. Rapid, secure, and reliable Hosting Solution. Learn more about );
In this instance, it will open an external site in an entirely new browser window as it can use the target="_blank"
and rel="noreferrer"
attributes.
Making components for Gatsby
Gatsby's modular architecture enables users to create reusable building elements for your web pages. Instead of duplicated code across several pages, you are capable of encapsulating the elements you use in your everyday work into parts, making your codebase more flexible, manageable, and productive.
If the code you use to display your website's homepage contains the navigation section, the main content, as well as a footer:
Import React using'react Import Link from 'gatsby' export default function home() Return ( home to ="/">Home to="/about">About to="/blog">Blog Take advantage of Static Web Hosting with StSH. Rapid, secure, and reliable Hosting Solution. Read more Hosted with by 's' ' href="https://.com/static-site-hosting"> Static Site Hosting . > );
Imagine having to copy the footer and navbar codes for each page of your site. Components can come in. You can create reusable components that can be used for the footer, navbar and any other component of code that could be used across various components and web pages.
For you to use components from Gatsby to use these components, you must make components folders in Gatsby. Create components folders in Gatsby. Create a component folder in the src folder. This will store all components. Next, create your components, e.g. Navbar.js and Footer.js. In the Navbar.js file, break the code into the following manner:
Import Link to 'gatsby', import React using'react";const Navigationbar () = return ( Home About Blog ) Export default Navbar
Additionally, Footer.js:
import React from 'react'; const Footer = () => return ( Hosted with by 's' ' href="https://.com/static-site-hosting">Static Site Hosting . ); ; export default Footer;
You can then add your documents to your pages or components and utilize this method:
import React from 'react'; import Navbar from '../components/Navbar'; import Footer from '../components/Footer'; export default function Home() return ( Enjoy Static Site Hosting With StSH. Fast, Secure, Reliable Hosting Solution. Learn more about );
Designing Layout Components
The most common manner of conduct in the development of websites is the creation of an layout component that reflects the overall style of the site. The layout component usually includes elements that are displayed in every page, such as the header, footer and navigation menus, sidebars and so on.
Make a new file called Layout.js in your component's /src/components directory. Then, you can determine the layout's format. This guide will define that the layout's design should comprise the footer and the navbar
import React from 'react'; import Navbar from './Navbar'; import Footer from './Footer'; const Layout = ( children ) => return ( className="content">children ); ; export default Layout;
Utilizing this component for layout is used, we use the components to wrap the contents of pages (provided as the children
). If you want to utilize the layout component in your pages, you have to import it and wrap your page contents with it. As an example, on the index.js page:
import React from 'react'; import Layout from '../components/Layout'; export default function Home() return ( Enjoy Static Site Hosting With StSH. Quick, secure and reliable Hosting Solution. Learn more );
By using a layout component will ensure that you have a consistent design and layout throughout your web pages, while also maintaining your code organized as well as up-to-date. This is a great way to manage the most common elements of your website efficiently.
Styling Pages and other components in Gatsby
The way you style your Gatsby site can be more adaptable as it lets you use different approaches like basic CSS and CSS-in-JS and CSS preprocessors, such as Sass. We'll show you how to design simple and mod-styled sites.
CSS Styling
In Gatsby there is a way to make an CSS file. You can then link it to whatever website or part you'd like to link it to and the file should work flawlessly. In this case, for instance, you can make an the styles folder within the the src folder, and then make global.css. global.css file with the CSS code.
For example, here are some common styles of components that were created earlier:
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500&display=swap'); * margin: 0; padding: 0; box-sizing: border-box; body background-color: #ddd; font-family: 'Poppins', sans-serif; width: 1200px; margin: 0 auto; a text-decoration: none; img width: 100%; nav display: flex; justify-content: space-between; height: 200px; align-items: center; nav .logo-img width: 100px; nav .nav-links a padding: 0 20px; font-size: 18px; @media (max-width:700px) body width: 100%; padding: 0 20px; nav .nav-links a padding: 0 18px; .footer width: 100%; text-align: center; margin: 100px 0 20px;
Then, you can add the CSS file into the components that you would like to style. In this instance and then you are able to add it to your Layout component to ensure it is applied to all components.
import React from 'react'; import Navbar from './Navbar'; import Footer from './Footer'; import '../styles/global.css'; const Layout = ( children ) => return ( className="content">children ); ; export default Layout;
Module CSS Styling
CSS Modules permit you to scope your styles to specific elements or even pages. This prevents clashes between styles, and also makes it easier to manage your CSS code. In the styles folder, create your CSS modules with the structure (pageName>.module.css and add the specific style into the files.
For instance, create home.module.css for the homepage, and include the following code:
.home_hero display: flex; justify-content: center; align-items: center; flex-direction: column; text-align: center; .home_hero h1 font-size: 60px; width: 70%; .home_hero p color: #6E7076; font-size: 20px; .btn background-color: #5333ed; padding: 20px 30px; margin-top: 40px; border-radius: 5px; color: #fff; @media (max-width:700px) .home_hero h1 font-size: 40px; .home_hero p font-size: 16px;
For you to utilize module CSS styles on your Gatsby website or other components to use the CSS module, you need to import the CSS style styles of the CSS module as an object on the top of your file or page and then use them in the following manner:
import React from 'react'; import Layout from '../components/Layout'; import * as styles from '../styles/home.module.css'; export default function Home() return ( Enjoy Static Site Hosting With StSH. Fast, Secure, Reliable Hosting Solution. Read more );
Utilizing Static Files in Gatsby
In Gatsby static files are referred to the assets such as pictures, fonts and CSS files as well as many other assets that are sent directly to the client's web browser without the need for server-side processing. These files are added to the the static directory within the root directory of your project.
For example, if you post the image -logo.png to the directory in the static directory, it will show in your component as follows:
link import from 'gatsby';import React from 'react';const the Navbar () = return ( to ="/"> home to ="/">Home to="/about">About to="/blog">Blog ) Export default Navbar
Gatsby will convert these relative paths to the proper URL after your site is built. Further in this tutorial, you will learn how to make images more efficient in Gatsby.
Integrations and Plugins
Gatsby offers a wide range of plugins, which allows you to extend the features of. There are plugins that can assist in SEO, analytics images, markdown optimization, image transformation, and much more. Configuring and installing plugins is simple and could significantly increase the performance of your site.
In this video tutorial, we will use four plug-ins:
- gatsby-transformer-remark: This plugin allows you to transform Markdown files into HTML content, making it easy to create and manage blog posts, documentation, or any text-based content.
- gatsby-transformer-sharp and gatsby-plugin-sharp: These plugins work together to optimize and manipulate images in your Gatsby project.
- gatsby-source-filesystem: This plugin enables you to source files from your project directory and make them available to query with GraphQL.
To install these plugins utilized in your Gatsby project, execute the following command within the project's root directory for installation:
npm install gatsby-transformer-remark gatsby-transformer-sharp gatsby-plugin-sharp gatsby-source-filesystem
You can then modify them within your gatsby-config.js file. Here is an example of the way you can set up the plug-ins
module.exports = plugins: [ // ...other plugins // Transform Markdown files into HTML 'gatsby-transformer-remark', // Optimize and manipulate images 'gatsby-transformer-sharp', 'gatsby-plugin-sharp', // Source files from your project directory resolve: `gatsby-source-filesystem`, options: name: `posts`, path: `$__dirname/src/posts/`, , , resolve: `gatsby-source-filesystem`, options: name: `images`, path: `$__dirname/src/images/`, , , ], ;
Two gatsby-source-filesystem configurations are created, pointing to two folders: posts and images. Posts will store some markdown files (blog posts) that would be transformed with gatsby-transformer-remark, and images will store images for the blog and other images you wish to optimize.
Make sure to restart your local development server every time you modify the gatsby-config.js file.
Creating Blog Posts
Once we've installed our plugins create posts folders in the post folder within the src directory. After that, create two Markdown files with the following contents:
post-1.md:
--- title: "Introduction to Gatsby" date: "2023-10-01" slug: "introduction-to-gatsby" description: "Learn the basics of Gatsby and its features." featureImg: ../images/featured/image-1.jpeg --- Welcome to the world of Gatsby! In this article we'll walk you through the basic concepts of Gatsby as well as its shrewd features.
Also post-2.md:
--- title: "Optimizing Images in Gatsby" date: "2023-10-05" slug: "optimizing-images-in-gatsby" description: "Explore how to optimize images in your Gatsby project." featureImg: ../images/featured/image-2.jpeg --- Images play a crucial role in web development. In this blog we'll look at how to optimize images in Gatsby using plugins.
They are Markdown files contain frontmatter with metadata for blog posts, including names and dates as well as slugs, descriptions, and pictures.
For Gatsby, GraphQL can query the Gatsby novel.
When you run gatsby develop
in your terminal, you'll notice that, in addition to the link gatsby-source-filesystem, which opens your project on the web, you also see the http://localhost:8000/___graphql URL. This link gives access to the GraphiQL editor that you could use to create the development of your Gatsby project.
After you launch the editor, you will see the following interface:
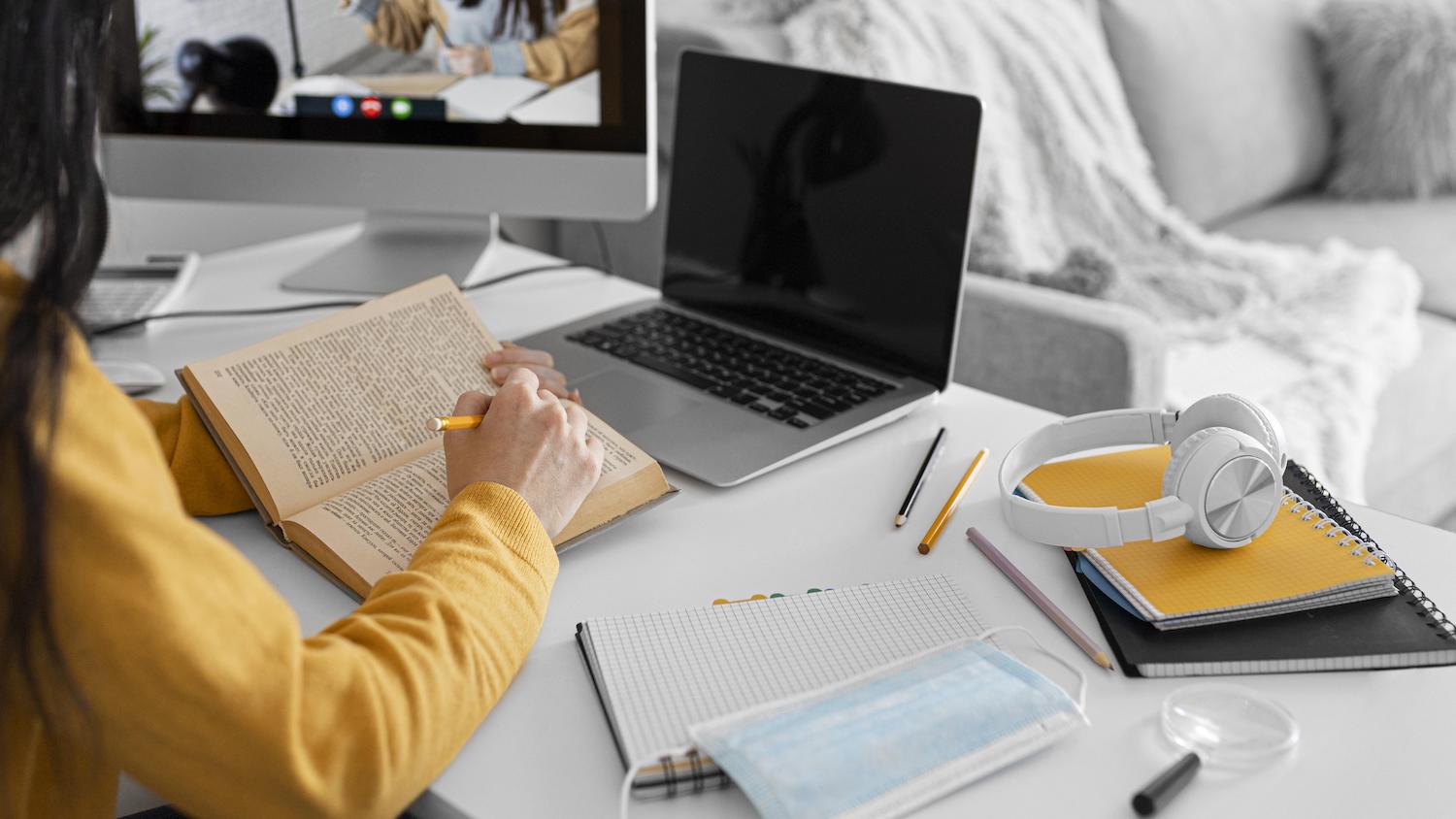
It's possible to obtain the most information regarding your site from this editor. It is because you've made markdown files, and have completed the settings in your gatsby-config.js file. It is possible to query the Markdown files and their content using the query below inside the editor
blogList query *
This query extracts data from all Markdown files using the allMarkdownRemark
. It extracts details such as the title
, slug
as well as descriptions
from the top of every Markdown file, along with their id
.
After you've written your query click after you've written your query then press the "Play" button (a right-facing triangular) to perform the query. Results will be displayed in the upper-right corner of the editors.
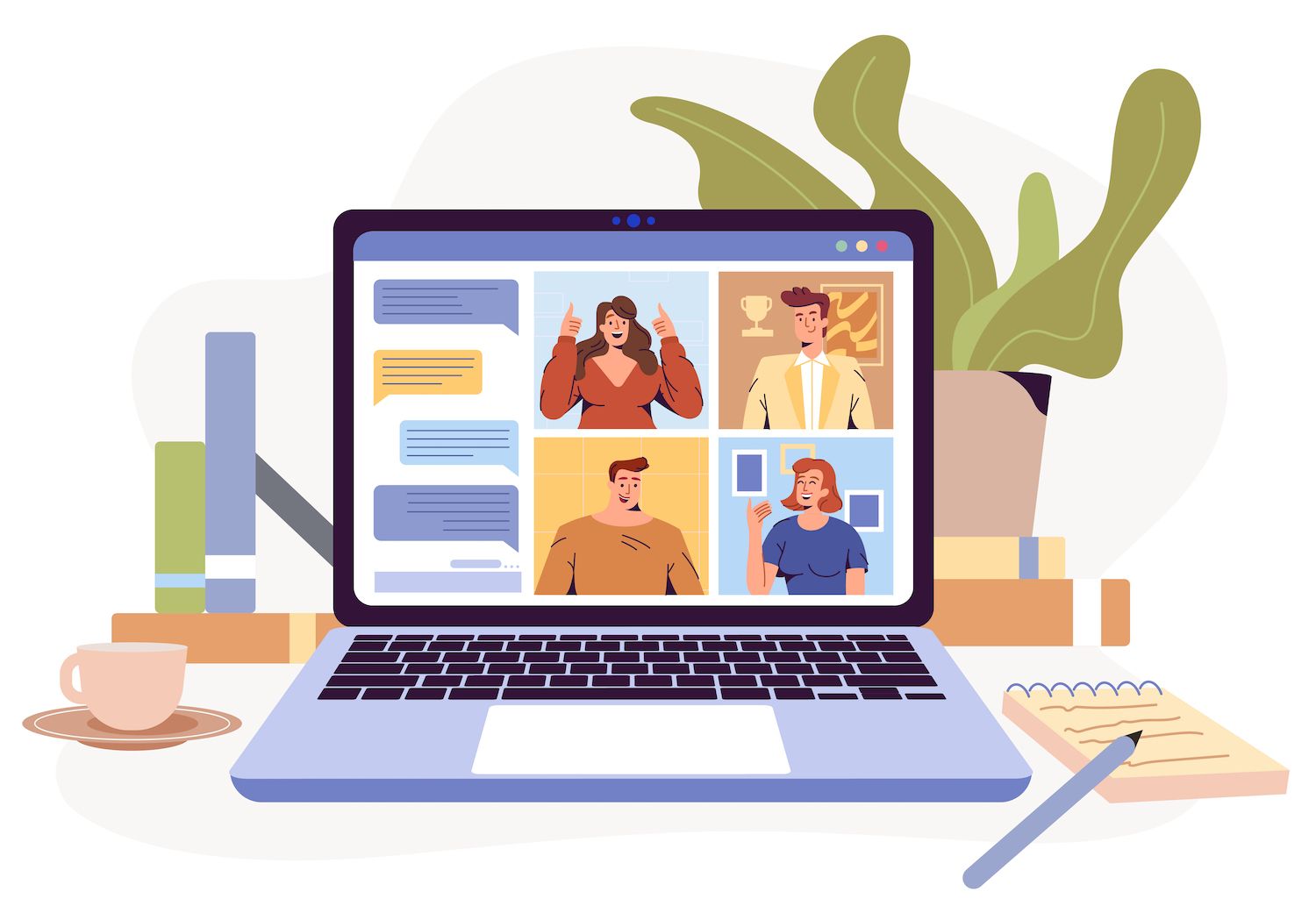
Then, you can make use of GraphQL to query the Markdown information within the pages of your component. To query this data blog/index.js or page blog/index.js page, first install graphql
from the gatsby program
. In the last line in your JSX code, include the following details:
export const query = graphql` query BlogList allMarkdownRemark nodes frontmatter title slug description id `;
The above code uses this graphql
tag to produce a GraphQL query. This is referred to by the name of queries
. The way you're putting in your blog/index.js file should be looking like is the following format:
import graphql, Link from 'gatsby'; import React from 'react'; import Layout from '../../components/Layout'; import * as styles from '../../styles/blog.module.css'; const blog = ( data ) => const posts = data.allMarkdownRemark.nodes; return ( Blog posts.map((post) => ( post.frontmatter.title post.frontmatter.description )) ); ; export default blog; export const query = graphql` query BlogList allMarkdownRemark nodes frontmatter title slug description id `;
In the code above in the above code, you utilize query
result using your details
prop inside your component. Then, you go through the posts'
data with your JavaScript method. Next, you can display each post's title in an order.
To prevent errors, make a blog.module.css file in the styles folder. Then, add the following code:
.blog_cont h2 font-size: 40px; margin-bottom: 20px; .blog_grid display: grid; grid-template-columns: 1fr 1fr 1fr; gap: 20px; @media (max-width:700px) .blog_grid grid-template-columns: 1fr; .blog_card background-color: #bfbfbf; padding: 20px; border-radius: 5px; color: #000; transition: all .5s ease-in-out; .blog_card:hover background-color: #5333ed; color: #fff; .blog_card h3 margin-bottom: 15px; .blog_card p margin-bottom: 15px;
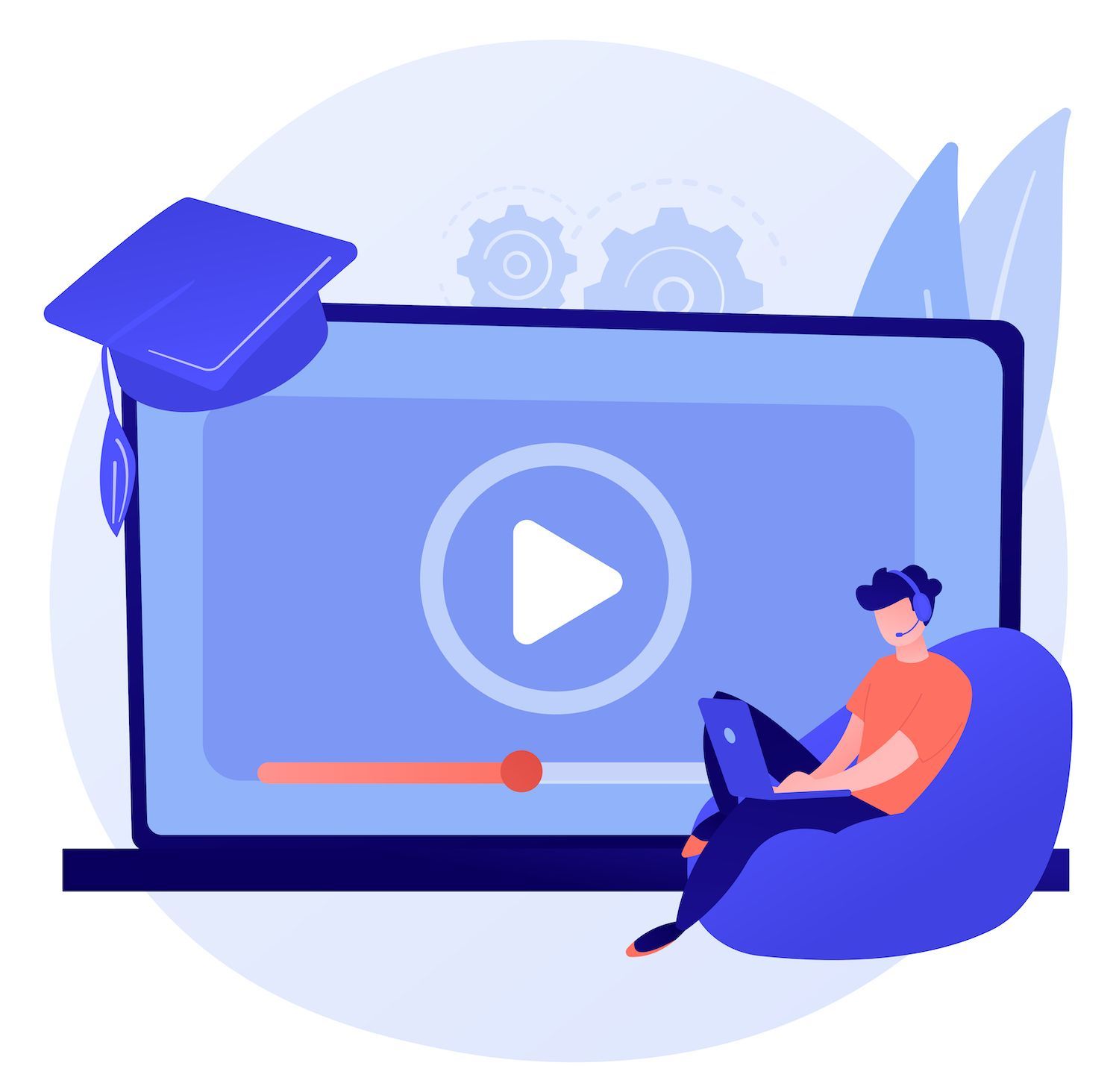
Lerner's Templates, and Generating dynamic pages in Gatsby By Using GraphQL
in Gatsby the Gatsby, templates and dynamic websites are the primary principles that let you create dynamic and flexible websites that are driven by data. Templates let you define the design and structure of your webpages. GraphQL helps you to gather information to fill these templates automatically.
Making a template for Blog Posts
Imagine that you wish to create a blog where each blog post blog is the same in structure that includes an introduction and contents. You can create a BlogDetails
template for this design. Within the the src folder, you can build a template folder. Then, create blog-details.js file: blog-details.js file:
import React from 'react'; import Layout from '../components/Layout'; import * as styles from '../styles/blog-details.module.css'; const BlogDetails = () => return ( Title className=styles.html dangerouslySetInnerHTML= /> ); ; export default BlogDetails; In this instance, the BlogDetails template defines the format for blog post. Next, let's utilize GraphQL to retrieve data from particular blog posts, and then pass it as props to the template. Making Dynamic Pages to make dynamic websites, you need to create the gatsby-node.js file within the root directory of your project. The file allows users to define how the pages are produced. The gatsby-node.js file, utilize GraphQL to get the data that you wish to utilize for dynamic websites. In the case of, for instance, if you've Markdown blog posts, you can query their slugs: const path = require(`path`); exports.createPages = async ( graphql, actions ) => const data = await graphql(` query Articles allMarkdownRemark nodes frontmatter slug `); data.allMarkdownRemark.nodes.forEach((node) => actions.createPage( path: '/blog/' + node.frontmatter.slug, component: path.resolve('./src/templates/blog-details.js'), context: slug: node.frontmatter.slug , ); ); ; In this example in this example, we search the slugs for all Markdown posts and create dynamic pages for each article making use of this BlogDetails template. The context object can be used for providing data to the template. The information (slug) is what the template uses to obtain other information which is aligned with the data in the slug. We must first know how image optimization works in Gatsby prior to adding the GraphQL query to the template's web page. Image Optimization in Gatsby Earlier, you installed and configured the gatsby-transformer-sharp and gatsby-plugin-sharp along with gatsby-source-filesystem for sourcing images. With these plugins, it is possible to optimize and query images using GraphQL. Here's an example of how to query and display an optimized image using the gatsby plugin-sharp: export const query = graphql` query file(relativePath: eq: "example.jpg" ) childImageSharp fluid ...GatsbyImageSharpFluid `; In the code above in the above code, you're requesting an image named example.jpg from the images source and then using the fluid property of the image to show it in a the most responsive and optimized rendering. You can then load the image in images-gatsby for optimized images. import React from 'react'; import graphql from 'gatsby'; import Img from 'gatsby-image'; const ImageExample = ( data ) => const fluid = data.file.childImageSharp; return ( ); ; export default ImageExample; Queuing Dynamic Pages Gatsby is using the template to build distinct pages for every blog post. Let's now include a GraphQL query on the template page to fetch the relevant data, based on the URL: import graphql from 'gatsby'; import Img from 'gatsby-image'; import React from 'react'; import Layout from '../components/Layout'; import * as styles from '../styles/blog-details.module.css'; const BlogDetails = ( data ) => const html = data.markdownRemark; const title, featureImg = data.markdownRemark.frontmatter; return ( title ); ; export default BlogDetails; export const query = graphql` query ProjectDetails($slug: String) markdownRemark(frontmatter: slug: eq: $slug ) html frontmatter title featureImg childImageSharp fluid ...GatsbyImageSharpFluid `; In the above code, you will notice it is querying for the optimized image and querying for the blog entry that matches the slug. Check out the complete Source code for the Gatsby project at GitHub. Install Gatsby static websites When you have completed your repo, take these steps to install your static website : Sign in or create an account in order to view your dashboard on your My Dashboard. Authorize the Git account with your provider. Click on Static Sites in the sidebar left, then choose Add Site. Add Site. Choose the repository that you would like to be deployed from, as well as the branch you wish to use to deploy to. Assign a unique name to your site. My will detect the build parameters that are used for the Gatsby project and automatically. You will see the below configurations already set for you: Command to build npm run build Node version: 18.16.0 Publish directory: public Click on the Make a Site option.. Summary The book explains the basic concepts of routing and data source and the style of images, the image optimization plugins, deployment, and much more. Gatsby's performance, versatility and wide-ranging ecosystem make it an ideal choice to create static sites. Whether you're creating an individual blog, portfolio website or even a corporate site, Gatsby has you covered. Now it's time to put that skills to use and create your personal Gatsby website. Did you use Gatsby for building anything? Do not hesitate to discuss your experiences and projects to us via the comments in the box below. Joel Olawanle Joel is an Frontend developer at the company the Technical Issues Editor position. Issues. He is a passionate educator with a love of open source software. He has written over 200 technical articles, mainly focused on JavaScript and the frameworks it uses. Website LinkedIn Twitter
This post was posted on here