Master state management by Vue.js employing Pinia (r) and Pinia (r)
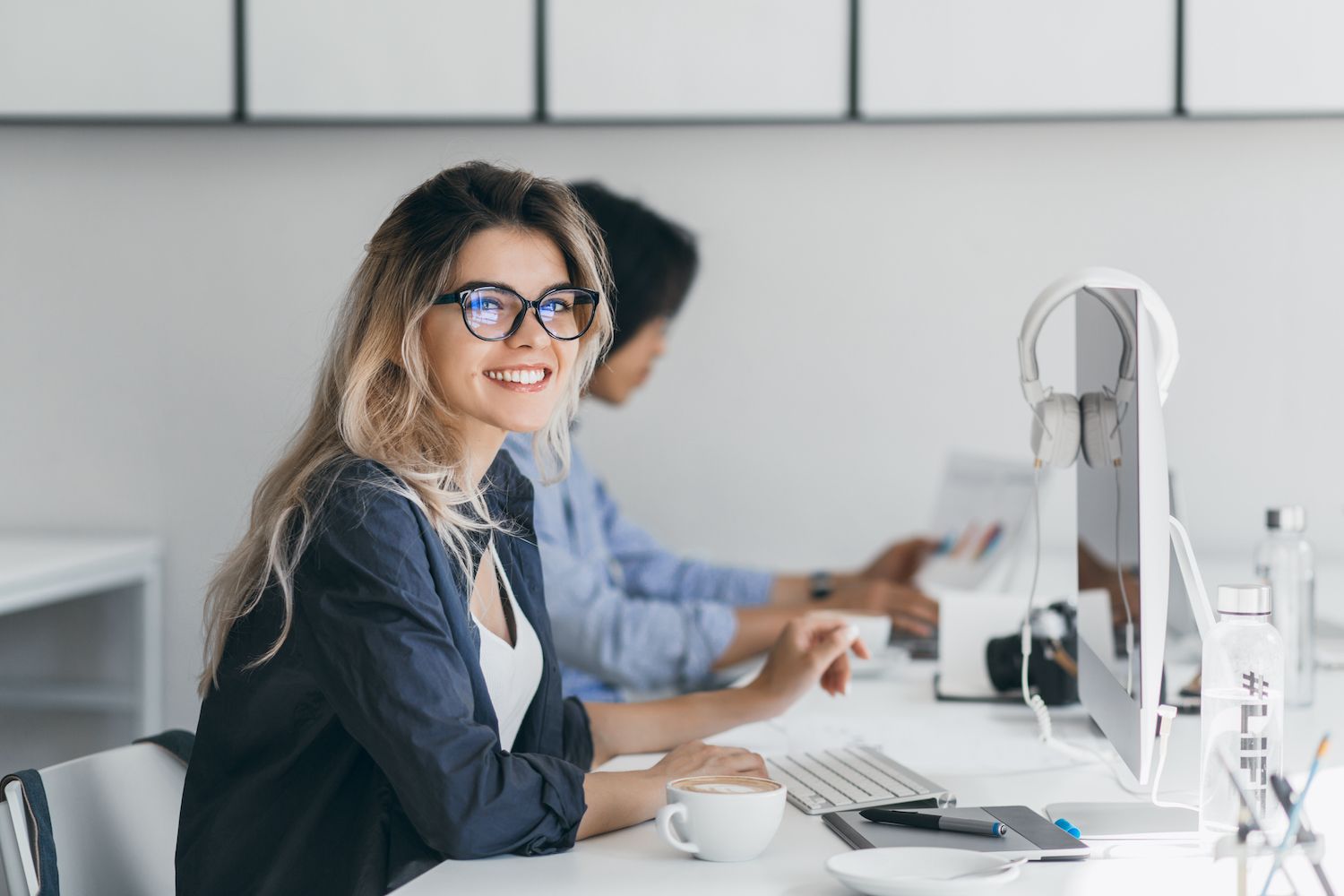
-sidebar-toc>
In Vue the state management feature is a key feature of Vuex which is a library that offers an centralized database of the various components utilized in applications. However, recent advancements within the Vue framework has led to Vuex's successor Pinia.
Pinia provides a lighter more modular, intuitive, and user-friendly way to manage data. It integrates seamlessly into Vue's Reactivity System and Composition API that allows developers to monitor and control the shared data in an incredibly flexible and manageable manner.
The action is in place: Pinia vs Vuex
A popular library to use to manage state in the Vue application, Vuex was a central repository that could be used by all application components. Since the advancements in Vue, Pinia represents a newer option. It's worthwhile to look at what makes it different from Vuex.
- API variants HTML0 API does have some distinctions the Pinia Composition API offers an simpler and intuitive API when compared with Vuex, making it easier to control the application's state. Furthermore, the design of its API is similar to Vue's Options API, familiar to many Vue developers.
- Typing support The past has witnessed many Vue developers have been dissatisfied with the lack of support for type in Vuex. However, Pinia is a fully managed by type which eliminates all concerns. The type safety feature helps to prevent errors during runtime, contributes in the readability of code, as well as facilitates easier scaling capability.
- Reactivity technology Both libraries use Vue's reactivity mechanism, however Pinia's approach aligns more closely in line with Vue 3. Its Composition API. Even though the API to reactivity can be effective however, managing the complex nature of state in big applications can be challenging. Simple and flexible architecture reduces the stress of managing state within the Vue 3 applications. Storage patterns within Pinia let users define an area of storage to manage a specific portion of the app's state, making it easier for the process of organizing it and sharing the data across different parts.
- Its slim designIts lightweight design - with just 1KB of memory, Pinia integrates seamlessly into your development environment. its lightweight weight could enhance the performance of your applications and loading times.
What is the best way to set up an Vue project with Pinia
In order to integrate Pinia inside Vue, you must first integrate it into the Vue project, initialize your project using Vue CLI as well as Vite. After the project has been initialized, you can install Pinia by using yarn or npm as dependencies.
- Create your own Vue project by using Vue CLI and Vite. After that, follow the instructions to set up your project.
// Using Vue CLI vue create my-vue-ap // Using Vite npm create vite@latest my-vue-app -- --template vue
- Switch your directory to the new project folder:
Cd my-vue app
- Install Pinia as a dependency on your project.
// Using npm npm install pinia // Using yarn yarn add pinia
- The main entry files (usually main.js or main.ts ), import Pinia and instruct Vue to make use of these:
import createApp from 'vue'; import createPinia from 'pinia'; import App from './App.vue'; const app = createApp(App); app.use(createPinia()); app.mount('#app');
With Pinia installed and set up within Vue, once you've installed and set up the Vue Project, you're now prepared to establish and manage the state-management stores.
How to create a store using Pinia
The store is the foundation of state management in the pinia powered Vue application. It aids you in managing all data across the entire application in a consistent and coordinated manner. The storage area is where you decide to define the data, manage it, and store any information that you would like to share with the other components of your application.
The centralization of your application is essential as it can organize the current state of your application and manages the flow of data, making it simpler to analyze and predict.
Additionally, a shop in Pinia is more than just a place to store the status of objects: its included features allow you to modify the state of things through actions as well as identify derived states with getters. These features built into the system help create a more efficient and maintainable codebase.
This illustration demonstrates how to create the fundamental Pinia store inside a project's src/store.js file.
import defineStore from 'pinia';export const useStore = defineStore('main' state) () (count: 0); (count: 0,), actions Thegetters: doubleCount (state) = state.count 2 );
What is the best way to get access to state stored in the components
Comparatively with Vuex, Pinia's approach to managing state access and administration is more comprehensible, particularly when you're already acquainted the Vue 3's Composition API. This API is a set of APIs which allow for the inclusion of reactive and compatible functions into your component.
Check out the code.
> import store from './store' and export the
The store
function grants access to the Pinia store. You can download it from store.js using import useStore from './store";
.
As a feature of Vue3's Composition API, the setting
function defines your component's reactive state and the purpose of it. In this method it is possible to Store()
to access the Pinia store.
After, const count = store.count
accesses the store's count
property, making it available to the component.
After that, setup
returns the number
and allows the template to display the count. Vue's reactivity system means that your template's design alters the value of count
as it changes in the store.
Below is a screen shot of the final results.
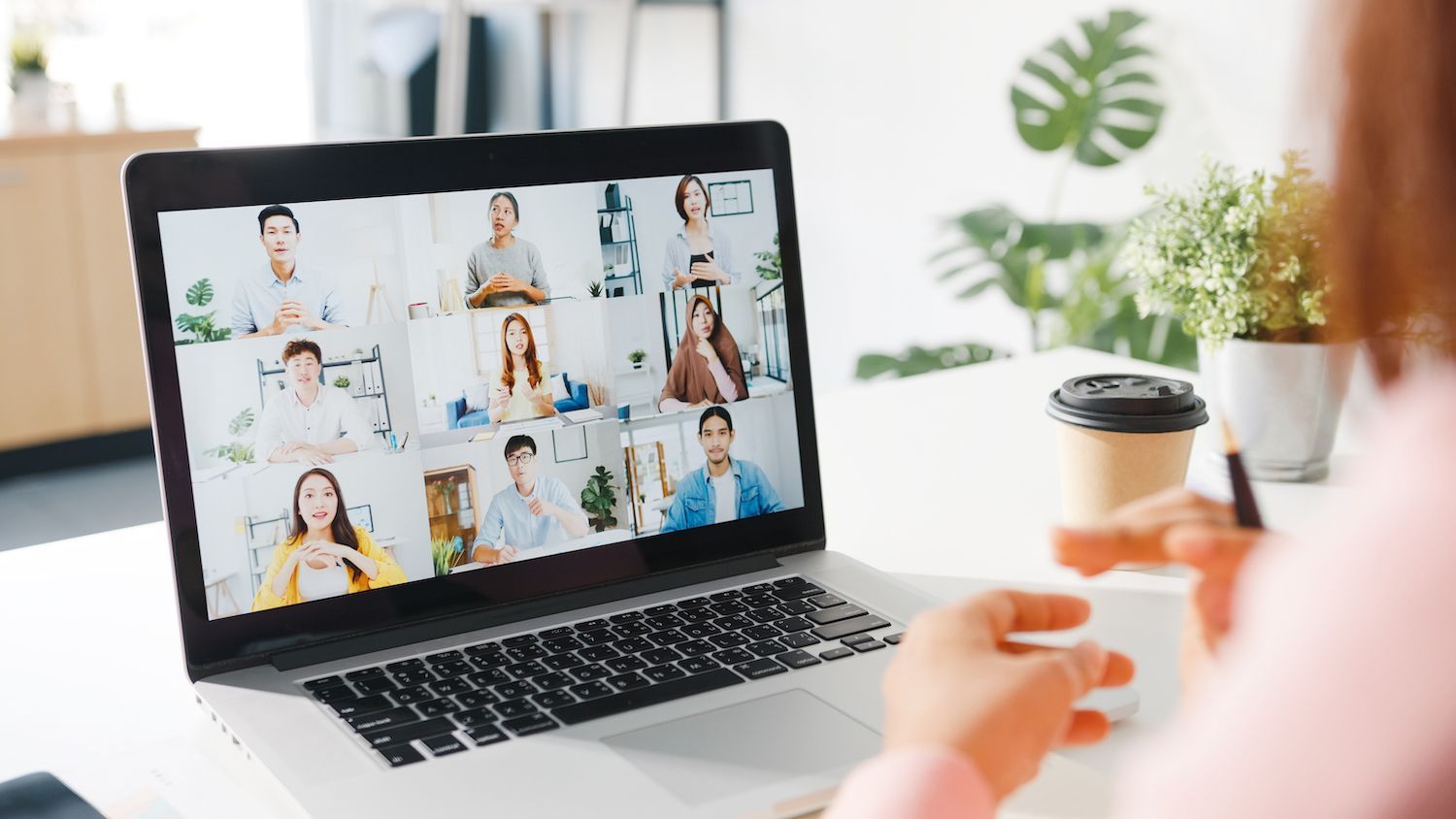
This image illustrates the benefits of Pinia:
- Simple It's Pinia gives direct access to the state of the store without using mapping functions. Contrarily, Vuex needs
mapState
(or similar tools) for the same access. - Direct store access direct store access Pinia allows you to access the state properties (like
store.count
) directly that allows the code to be more readable and comprehensible. However, Vuex often requires getters for access to the simplest properties. This adds complexity and hinders the ability to comprehend.
Modify state using Pinia
In Pinia it's possible to alter an item's state through actions that are more flexible than Vuex changes. Consider the following function which increments a the state's count
property:
store.increment() //Increases the number of stores
On the other hand the process of creating a Vuex alternative involves the creation of a mutation in addition to at least any of these actions:
action, and
The Pinia action as well as its replacement Vuex code demonstrate a major difference between the libraries' complex codes. We'll explore the differences in greater detail.
- Indirect or Direct state change The
increment
actions demonstrates, Pinia actions directly mutate the store's state. In Vuex it's possible to change the state using mutations and make by taking actions. The separation between the two processes assures that the changes are tracked, however it is more complex and rigid than similar Pinia actions. - Asynchronous and synchronous methods While Vuex mutations remain synchronous and do not contain asynchronous process, Pinia actions can contain simultaneously synchronous as well as asynchronous codes. It is possible to perform API calls and other synchronous actions right inside actions, making for the smallest and most concise code base.
- Simple syntax, Simplified syntax Vuex generally requires the definition of changes and steps required for making them occur. Pinia can eliminate this requirement. Its capability to modify the state within actions reduces the need for boilerplate and makes your code current. In Vuex simple state updates require specifying an action and a mutation, even if the action is just doing the transformation.
The change from Vuex and then Pinia
Transitioning to Pinia will bring many advantages with regards to simplicity, flexibility and maintainability, but it requires thoughtful planning and consideration in order to ensure a smooth transition.
Prior to making the change, ensure that you
- Be familiar with the distinctions between Pinia's and Vuex's architecture states management patterns, APIs and state-of-the art. It is crucial to understand these distinctions in order to refactor your code to take full benefit of the features that are offered by Pinia.
- Restructure and examine your Vuex state, action change, and getters to fit into Pinia's structure. Keep in mind that within Pinia you can declare state as a function. You can directly alter states by taking actions, and also implement getters more smoothly.
- Think about how you can move into a Vuex module store. Pinia utilizes modules in not the same way as Vuex However, it is possible to set up your store for similar purposes.
- Examine your test procedures to adapt to changes in the management of state. This could mean updating how you mock stores or actions in your integration test and unit.
- Take note of how the change will affect the project's structure and organizational process. Pay attention to things like the name conventions and also the way you manage and integrate the stores across different components.
- Verify that your library works with the other Libraries. Ensure compatibility with the other. Make sure to check for updates or dependency changes which could impact.
- Review any change in performance. Pinia is usually lighter than Vuex but it's essential to keep an eye on your application's performance during as well as after any change to ensure that there aren't issues.
Converting a store that was developed by Vuex to Pinia will require a number of actions to modify their structure and API. Consider the Pinia store that was originally.
The store identical to the one in the Vuex store.js file appears as follows.
import Vue from 'vue'; import Vuex from 'vuex'; Vue.use(Vuex); export default new Vuex.Store( state: count: 0, , mutations: increment(state) state.count++; , , actions: increment(context) context.commit('increment'); , , getters: doubleCount(state) return state.count * 2; , , );
Similar to the Pinia store too like the Pinia store, this Vuex instance also has a states
object. It has only an count
property. It can be set to zero
.
Its mutations
object is a class that can direct mutate state as well as the actions
object's methods commit the increase
transformation.
Getters object contains the doubleCount method. receiver
object contains an implementation of the doubleCount
method which multiplies each number
circumstance in the number of
and then returns the result.
The code in this example illustrates that the process of implementing stores in Pinia has a number of distinct distinctions from Vuex:
- Initialization -- Pinia doesn't require
Vue.use()
. - Structure -- In Pinia the state, it is the function that returns an object, allowing for greater TypeScript support as well as the ability to react.
- Actions Actions in Pinia are techniques that direct change the state, without modifications, which makes it easier to do the programming.
- Getters Getters HTML0Although they are similar to Vuex Getters in Pinia are defined within the definition of the store and allow direct connection to the state.
What are the best ways to use store's components in component stores?
If you're using Vuex It is possible to make use of the store in this way:
import mapGetters, mapActions from 'vuex'; export default computed: ...mapGetters(['doubleCount']), , methods: ...mapActions(['increment']), , ;
To utilize Pinia it's the situation, and it's a matter of:
> import useStore from '/src/store' and export the default configuration() const store = store() returning store, ; , ;
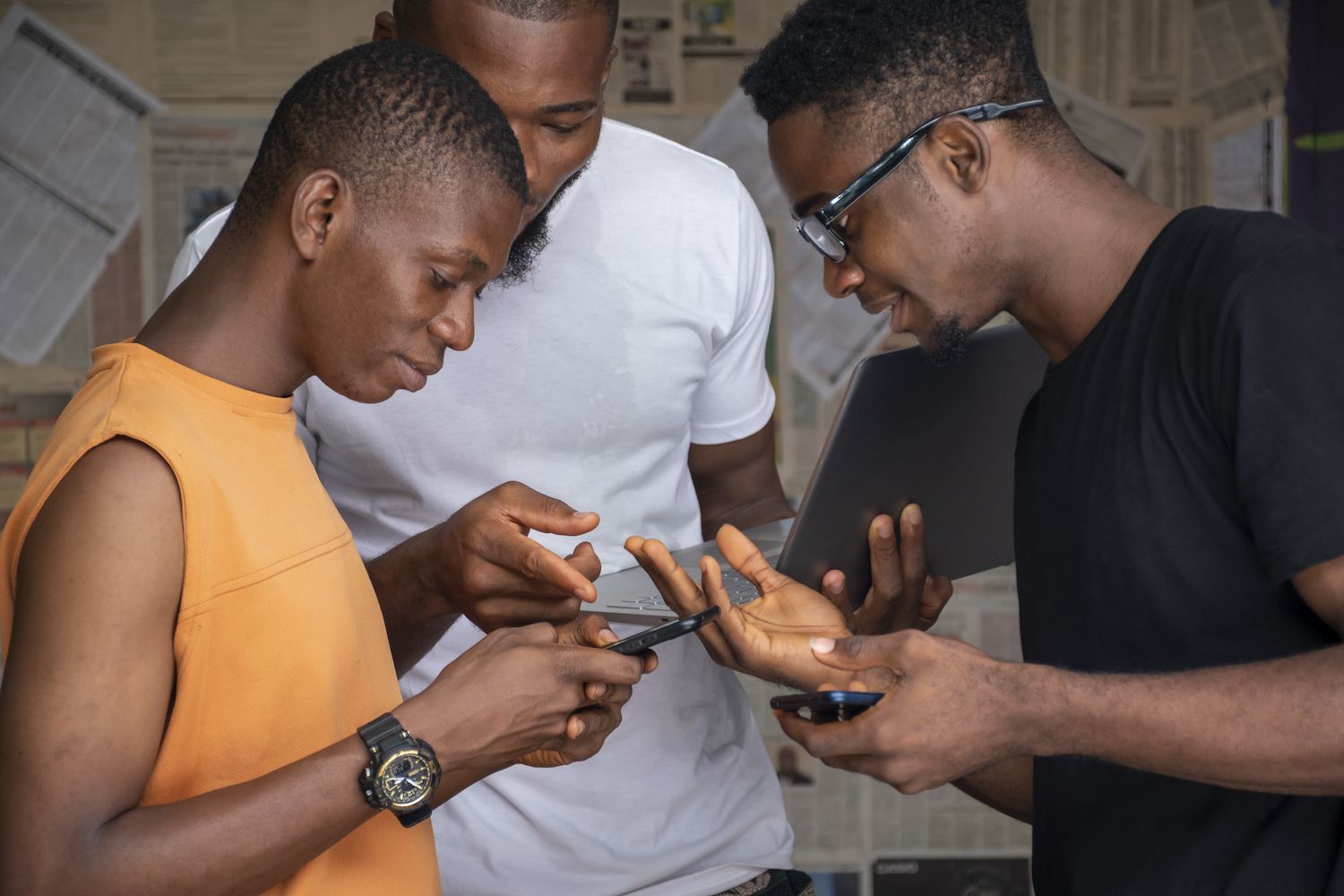
This example covers a basic conversion. If you wish to convert more intricate Vuex stores, especially ones which use modules, the conversion might be more thorough and redesigned to be more in line to the design of Pinia.
How to deploy your Vue application
Make your Dockerfile at your project's root and paste in the contents of this file:
From node:latest WorkDIR/app Copy package*.json .Run Installing NPM ./ . CMD ["npm", "run", "start"]
- Sign in or create an account to see Your dashboard. My dashboard.
- Autorize the Git service supplier.
- Select the App on the left sidebar, and then click the "Add App" button.
- The modal appears within the modal, choose the repository that you wish to use. If you are managing multiple branches, select the branch that you would like to utilize and provide a title for the application.
- Choose one of the data centers in the locations below.
- Choose the build environment you prefer, and then select the Dockerfile option in order to set the container image.
- If your Dockerfile isn't located in your repo's root directory, click Context to find its place and proceed. Continue.
- It is possible to make the beginning command field empty. Make use of
the npm command start
to begin your program. - Select the pod size and the number of times best suited to your requirements and Click on Continue.
- Complete the details of your credit card and click Create Application.
Summary
Moving from Vuex to Pinia is an important shift in the state of management within the Vue ecosystem. The ease of use and enhanced TypeScript support, as well as its compatibility in line with Vue 3.0's Composition API make the perfect alternative for today's Vue applications.
Jeremy Holcombe
The Content and Marketing Editor at , WordPress Web Developer, and Content Writer. Alongside all things WordPress, I enjoy playing golf, at the beach and watching movies. In addition, I'm tall and have issues ;).
Article was first seen on here