This is the process of building GraphQL APIs which make use of Node
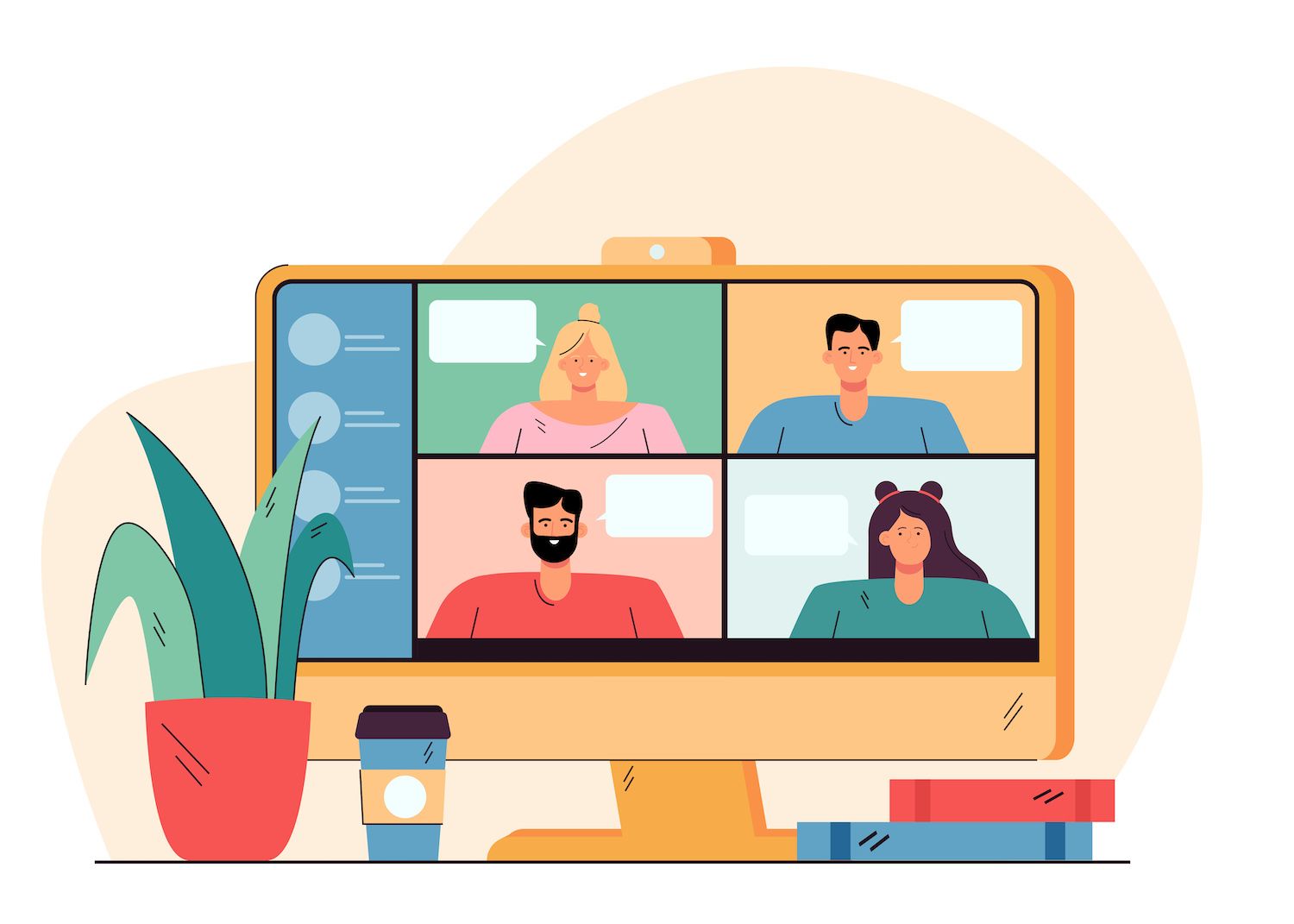
GraphQL is the most sophisticated technology utilized to build APIs. Although RESTful APIs are the preferred method commonly used to provide information to applications but they have some drawbacks, which GraphQL seeks to address.
GraphQL is the query language created by Facebook and was made an open source project in the year 2015. T offers a simple and flexible syntax which can be used to describe and access information using an API.
What exactly do you mean by GraphQL?
The official website: "GraphQL is a API query language and also as an API runtime which is able to assist in the creation of queries using your already-existing data. GraphQL provides a complete and simple description of the data that you offer through your API. Clients can get all the data they need and nothing less. It makes it simple to build APIs with a less time, and also gives developers the ability to use robust tools."
To build an GraphQL service, start by being able to define schema types, before creating fields based on these types of. Then, you create the function resolver that will run on each type and field whenever information needs to be gathered on the client's side.
The GraphQL Terminology
GraphQL type system is utilized to determine what information is able to be requested and the data which can be altered. This is at the heart of GraphQL. There are numerous ways to explain and change the data in the structure of GraphQ.
Types
GraphQL objects include fields with an element of solid. It is recommended to make 1-1 maps between models and GraphQL kinds. Here's an illustration GraphQL Type:
Type User Type Your User ID! "! "!" is the first name that is required. Stringlastname Email: username: String todos"[Todo"[Todo]" a completely distinct GraphQL kind
Queries
GraphQL Query defines all the queries that users have the ability to utilize to access the GraphQL API. Clients should build the base querythat encompasses all currently being queries running using the protocol.
It is then possible to determine the query, and match it to the RESTful API which has been tested and is compatible with the API:
Type RootQuery user(id ID) User # is matched to API/users/IDUsers The # matches API/users todostodo(id ID! ): Todo # Corresponds to GET /api/todos/:id todos: [Todo] # Corresponds to GET /api/todos
Mutations
If GraphQL is among the GET requests, then the changes include POST PUT, Patch, and the DELETE request, which alter the GraphQL API.
All changes will be combined into one RootMutation to show:
type RootMutation *
There is a chance that you already have used the -input types to specify the kinds of transformations that can be made, such as the input of users, TodoInput. It is always a good idea to determine the input type prior to altering or designing the original source.
There is a way to describe Input varieties like this:
Input UserInput: Firstname String! String for email with lastname! username: String!
Resolvers
Create a clean resolvers.js file and include the following code into it:
import sequelize from '../models'; export default function resolvers () const models = sequelize.models; return // Resolvers for Queries RootQuery: user (root, id , context) return models.User.findById(id, context); , users (root, args, context) return models.User.findAll(, context); , User: todos (user) return user.getTodos(); , // Resolvers for Mutations RootMutation: createUser (root, input , context) return models.User.create(input, context); , updateUser (root, id, input , context) return models.User.update(input, ...context, where: id ); , removeUser (root, id , context) return models.User.destroy(input, ...context, where: id ); , // ... Resolvers for Todos go here ; }
Schema
GraphQL schema determines the information GraphQL gives to the general public. Thus, all types of queries, types, and modifications are included in the schema, and available to all users.
Here are steps on how to make public the types of questions, and other changes that are available to the public:
schema query: RootQuery mutation: RootMutation
The script that we have written above, has been enriched by adding to it the RootQuery in the form of well as RootMutation that were originally designed to make them available all over the world.
What is GraphQL Do? Work With Nodejs and Expressjs
GraphQL is an programming language that is compatible with every programming language and Node.js isn't one of the languages. The official GraphQL website contains an article that describes JavaScript execution as well as the various versions of GraphQL which makes the writing process with GraphQL simple.
GraphQL Apollo offers an application for Node.js and Express.js and allows users to begin to learn about GraphQL.
This course will show the students how to develop and build your first GraphQL application using Nodes.js along with Express.js, the Express.js backend framework which incorporates GraphQL Apollo in the following section.
Configuring GraphQL By using Express.js
The process of building the GraphQL API server by using Express.js is a fantastic method for beginners to get started. In this tutorial, we'll show the steps to create an GraphQL server.
Make a fresh Project with Express
You can create a folder inside your project, and then install Express.js via the following instructions:
cd && npm init -y npm installation express
The command generates a new package.json file and it is installed with the Express.js library within the application you are running.
Are you interested in learning how we've increased the amount of people who visit us by 1,000?
Join more than 20,000 others who get our email every week. The emails are packed with unique WordPress details!
Our next step is to arrange our activities in manner as shown in the picture below. The program will consist of various modules in order to perform its functions like tasks, user, etc.
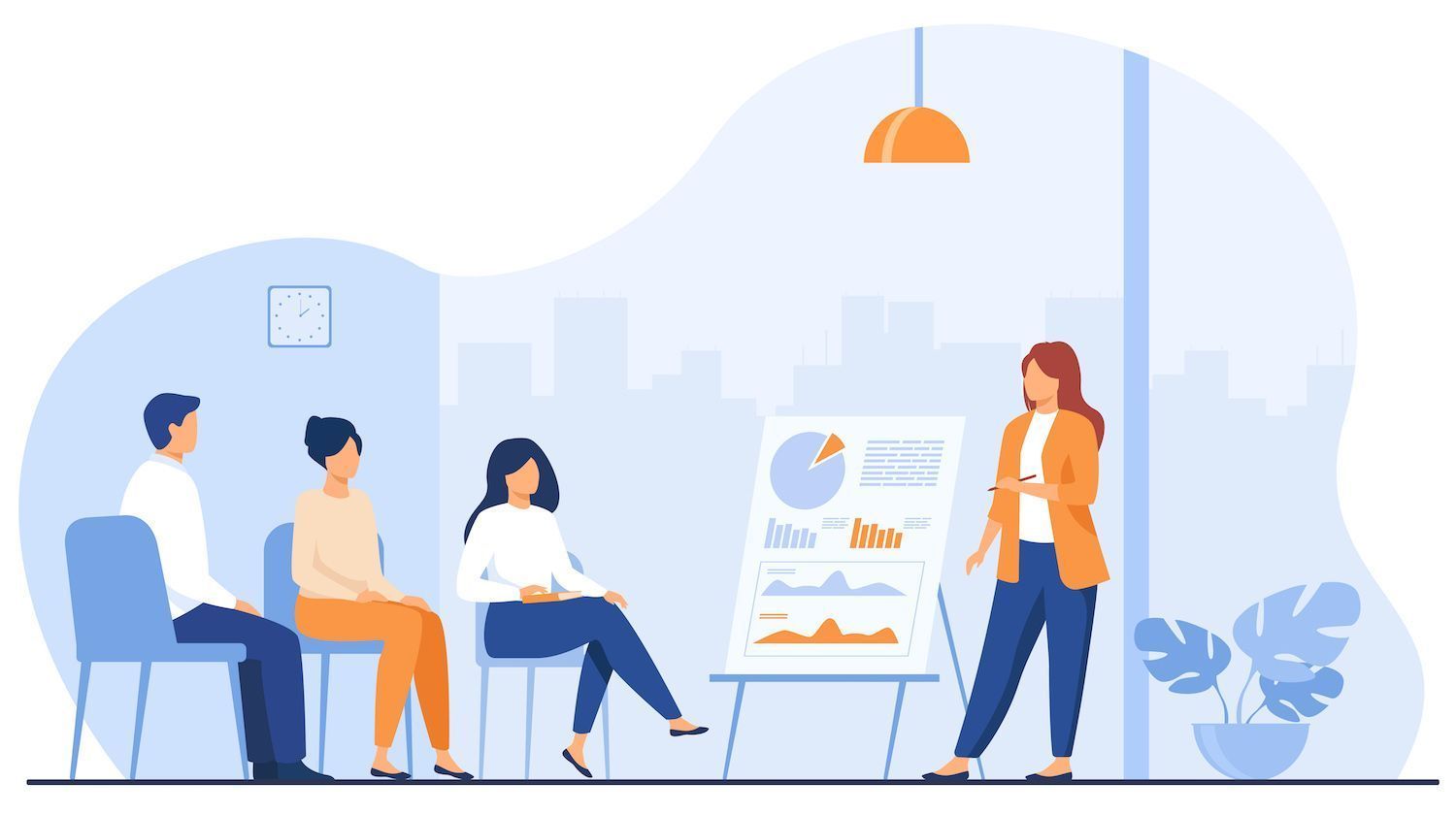
Initialize GraphQL
Set up your GraphQL Express.js dependency. The following command is needed to configure the installation
npm install apollo-server-express graphql @graphql-tools/schema --save
Method of creating Schemas and Types
The next step is to create an index.jsfile. index.jsfile inside the directory that contains modules. Then, we'll include this code block:
const gql = require('apollo-server-express'); const users = require('./users'); const todos = require('./todos'); const GraphQLScalarType = require('graphql'); const makeExecutableSchema = require('@graphql-tools/schema'); const typeDefs = gql` scalar Time type Query getVersion: String! type Mutation version: String! `; const timeScalar = new GraphQLScalarType( name: 'Time', description: 'Time custom scalar type', serialize: (value) => value, ); const resolvers = Time: timeScalar, Query: getVersion: () => `v1`, , ; const schema = makeExecutableSchema( typeDefs: [typeDefs, users.typeDefs, todos.typeDefs], resolvers: [resolvers, users.resolvers, todos.resolvers], ); module.exports = schema;
Code Walkthrough
Let's take a look at this code snippet for a closer look.
Step 1.
Then we download the required libraries, and then set up the most common types that include query and mutation. Both queries and mutations are an instance of GraphQL API as of now. But, we plan to extend the query and the mutations to support more schemas in the future.
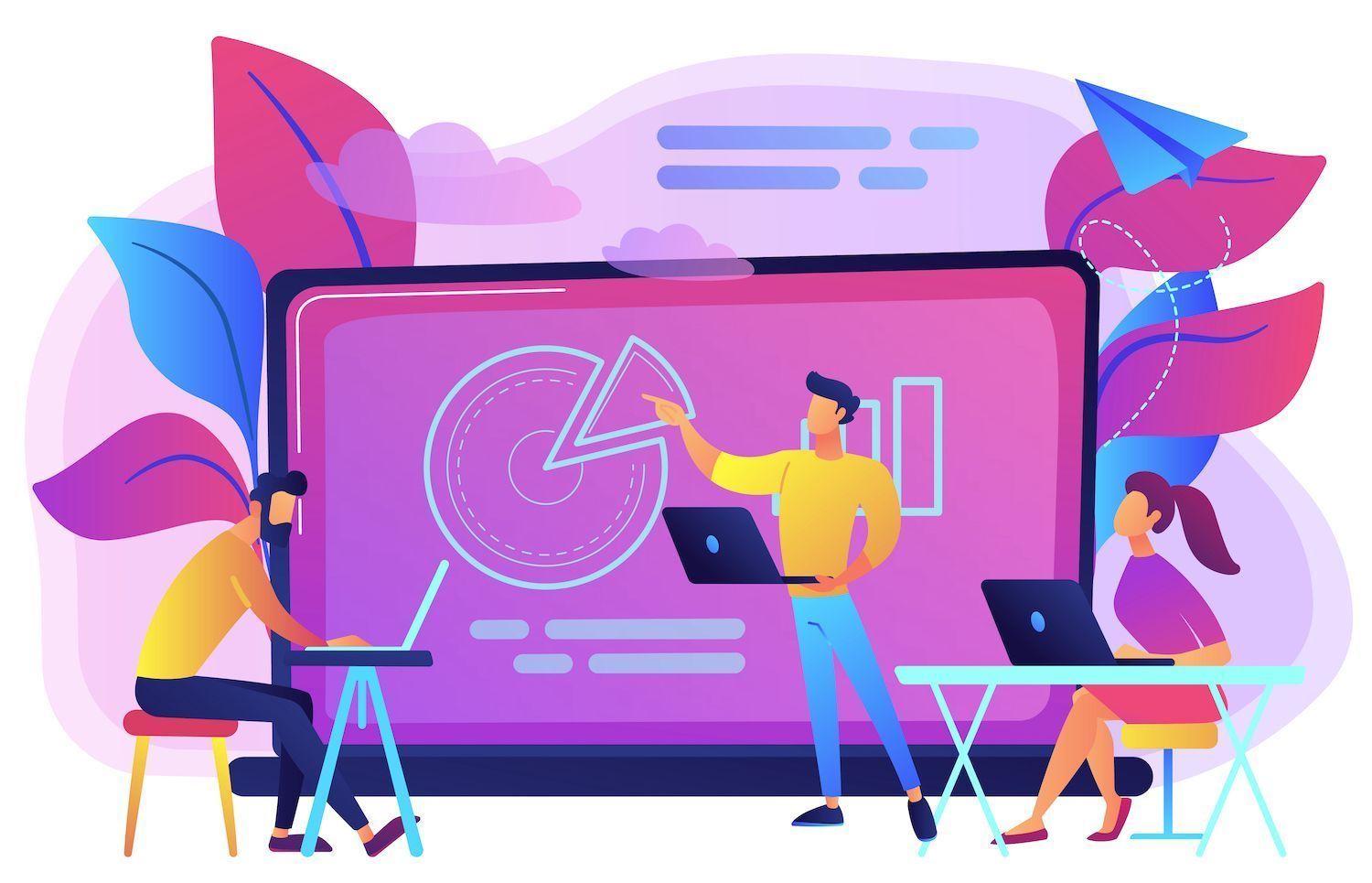
Step 2.
Then, we created a new type of scalar that can be described as a time-based representation and also our first resolver for the query, and the transform that we had before. Additionally, we generated a schema using this createExecutableEchema function.
The schema created includes schemas that we've taken in, and we will be adding further schemas when we develop them and eventually integrate them into the database.
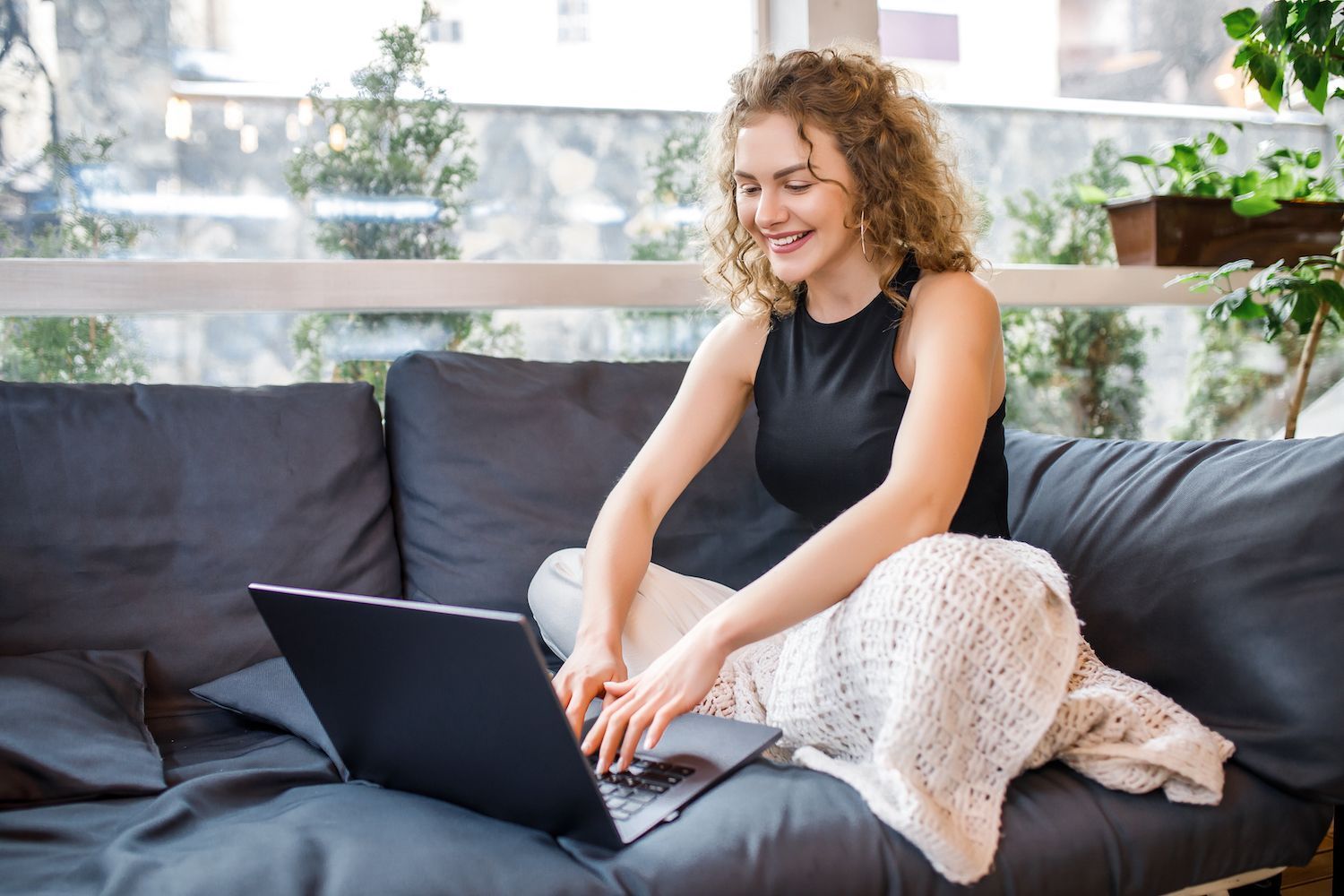
This example code shows how we have imported various schemas into the makeExecutableEchema method. This method helps to create an application which is well-organized to handle the complex problems. The next step is develop our own schemas that are built on Todo and the User schemas that we have developed.
Creating Todo Schema
The Todo schema describes the basic functions users of the app can perform. The schema below performs Todo operations , as well as CRUD.
const gql = require('apollo-server-express'); const createTodo = require('./mutations/create-todo'); const updateTodo = require('./mutations/update-todo'); const removeTodo = require('./mutations/delete-todo'); const todo = require('./queries/todo'); const todos = require('./queries/todos'); const typeDefs = gql` type Todo id: ID! title: String description: String user: User input CreateTodoInput title: String! description: String isCompleted: Boolean input UpdateTodoInput title: String description: String isCompleted: Boolean *do; // Implement resolvers for the schema fieldsconst resolvers = Resolvers to resolve queriesQuery such as todo, todos and// Resolvers for Mutations Mutation: createTodo, updateTodo, removeTodo, , ; Module.exports = typeDefs;module.exports = typeDefs, resolvers ;
Code Walkthrough
Let's work through this code-snippet. We'll break it down:
Step 1.
After that, we created structure that would allow us to control our work through the GraphQL forms, input and expanding. The keyword extension extensions keyword lets you create new queries, or modifications within your base query and also the change that the above-mentioned transformation has caused.
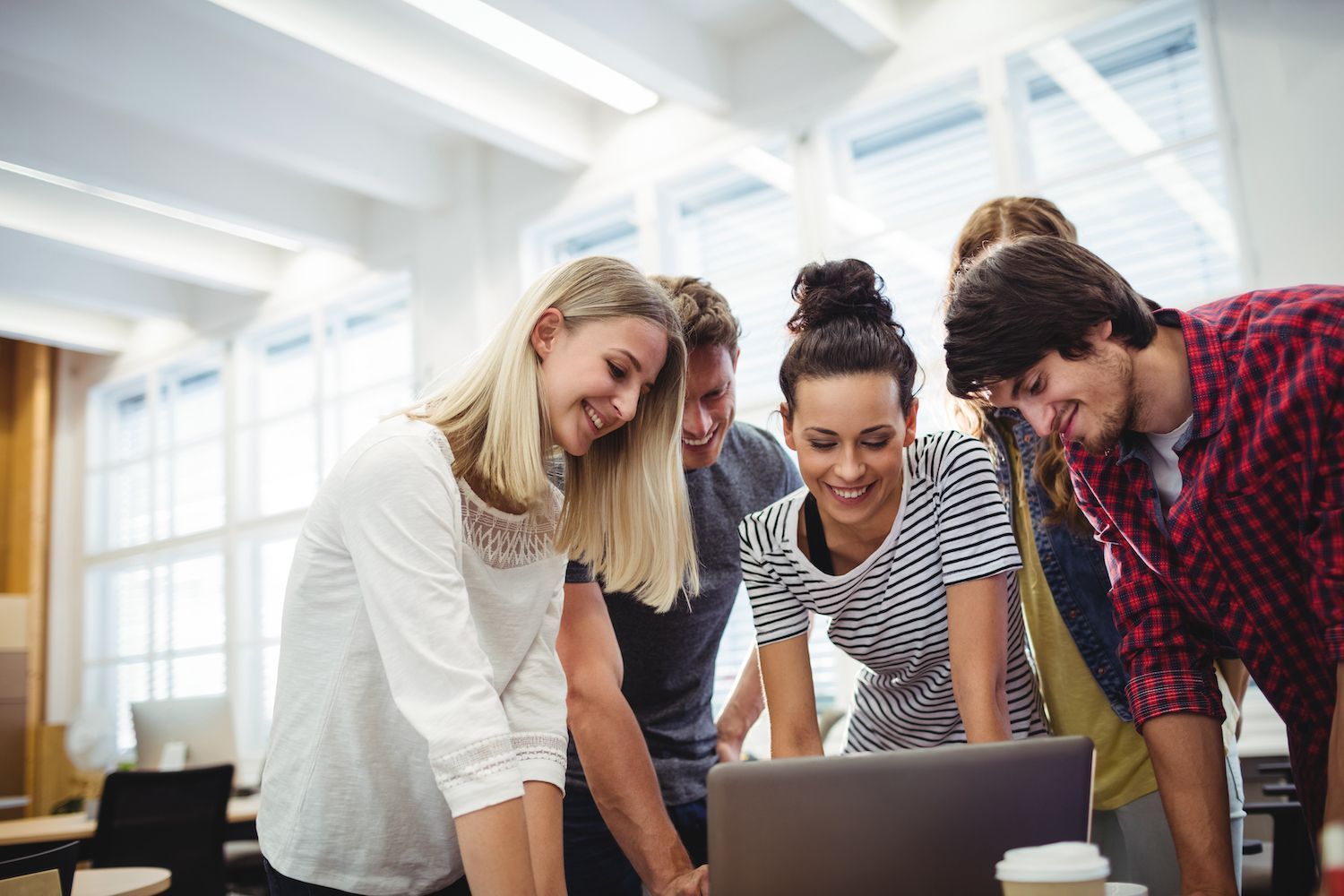
Step 2.
The resolver is one we designed, which will find the right data when an issue, or change is found to be.
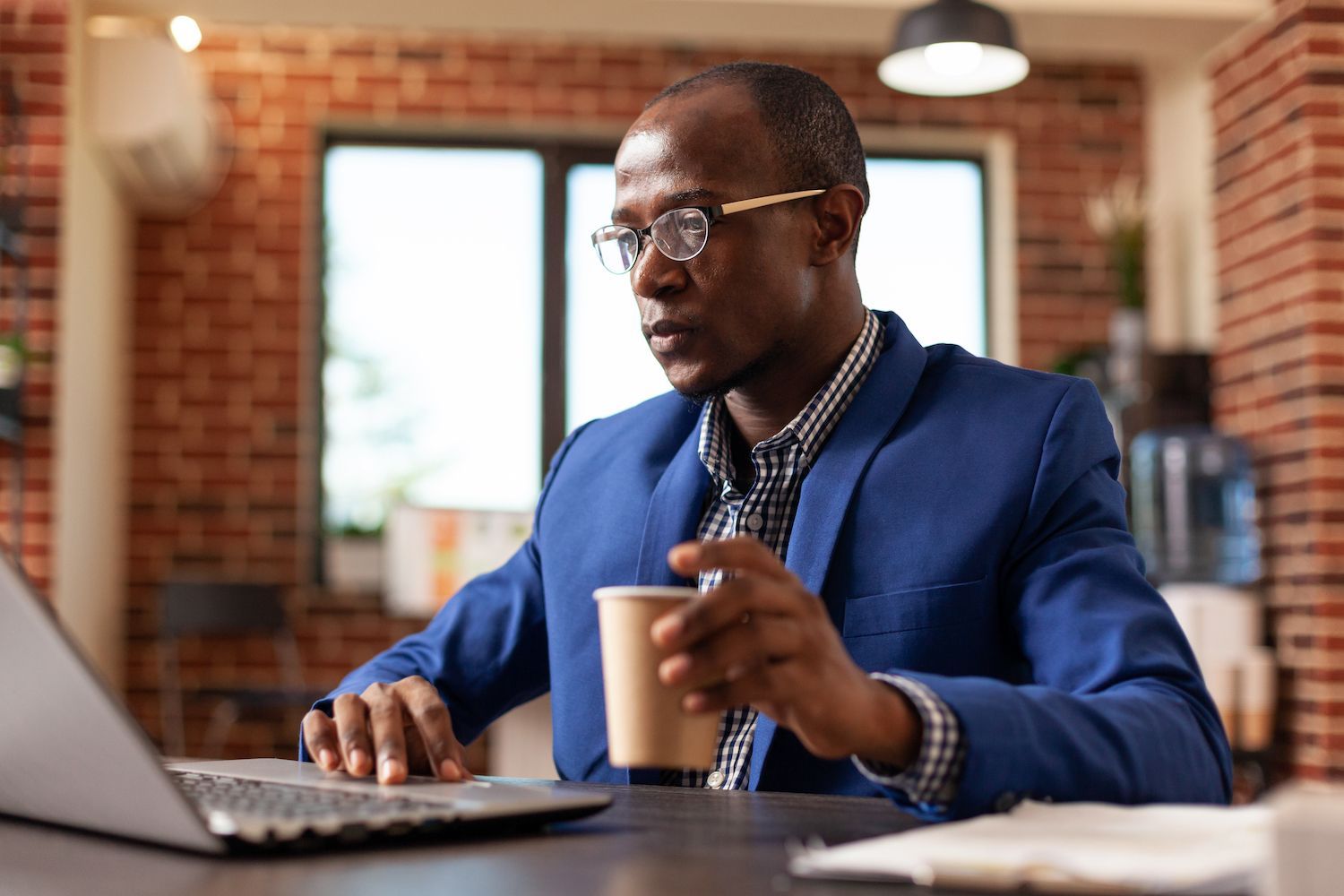
When we use resolver functions, it is possible to develop specific strategies that control the database and the business logic like in the create-todo.js example.
Create an create-user.js file in the ./mutations
folder and connect the business logic in the form of a new Todo within your database.
const models = require('../../../models'); module.exports = async (root, input , context) => return models.todos.push( ...input ); ;
This code provides an easy way to create the new Todo in our database with the ORM Sequelize. It is possible to learn more about Sequelize and how you can build it using Node.js.
It's possible to use the exact same process to create different schemas based on the specifics of your application, or transfer the entire application to GitHub.
The next thing to do is make a server using Express.js and to launch the latest Todo application using GraphQL in conjunction with Node.js
Configure and then start the Server
Lastly, we will set up our server using the apollo-server-express library we install earlier and configure it.
The apollo-server-express is a simple wrapper of Apollo Server for Express.js, It's recommended because it has been developed to fit in Express.js development.
Using the examples we discussed above, let's configure the Express.js server to work with the newly installed apollo-server-express.
Create the server.js file in your root directory. Transfer the server.js file in the root directory. Then copy this code:
const express = require('express'); const ApolloServer = require('apollo-server-express'); const schema = require('./modules'); const app = express(); async function startServer() const server = new ApolloServer( schema ); await server.start(); server.applyMiddleware( app ); startServer(); app.listen( port: 3000 , () => console.log(`Server ready at http://localhost:3000`) );
The code you have observed above signifies you've succeeded in completing the steps of creating your very first CRUD GraphQL server for Todos as well as Users. You can start your development server and access the playground using http://localhost:3000/graphql. If everything goes as planned then you will see the following display that appears as follows:
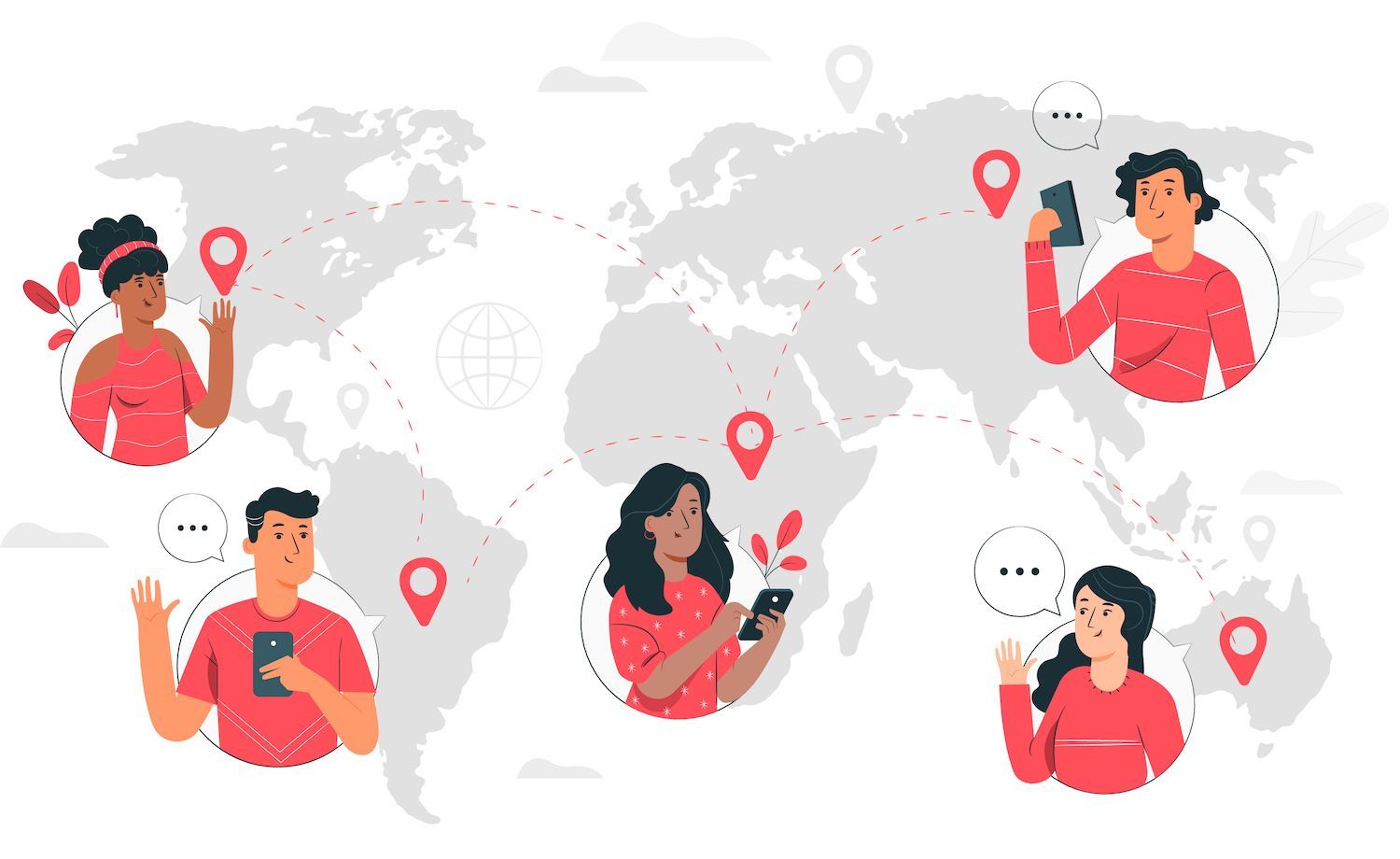
Summary
GraphQL is a cutting-edge technology used by Facebook which assists in the process of developing APIs with a large scale with RESTful architectural design.
This article provides an introduction to GraphQL and demonstrated how you can create your own custom GraphQL API using Express.js.
Let us know about projects you've created making use of GraphQL.
- Simple to create and manage My dashboard. My dashboard
- Support is available 24 hours a day.
- The best Google Cloud Platform hardware and network are powered by Kubernetes for the highest capacity
- Premium Cloudflare integration to improve speed as well as security
- Join the world's largest audience using more than 35 data centers and more than 275 POPs throughout the world.
The original post appeared this site
This article was originally posted this site
This post was posted on here