Use the best practices to play playing better. Your game should be completed before the 9th day of December 2022.
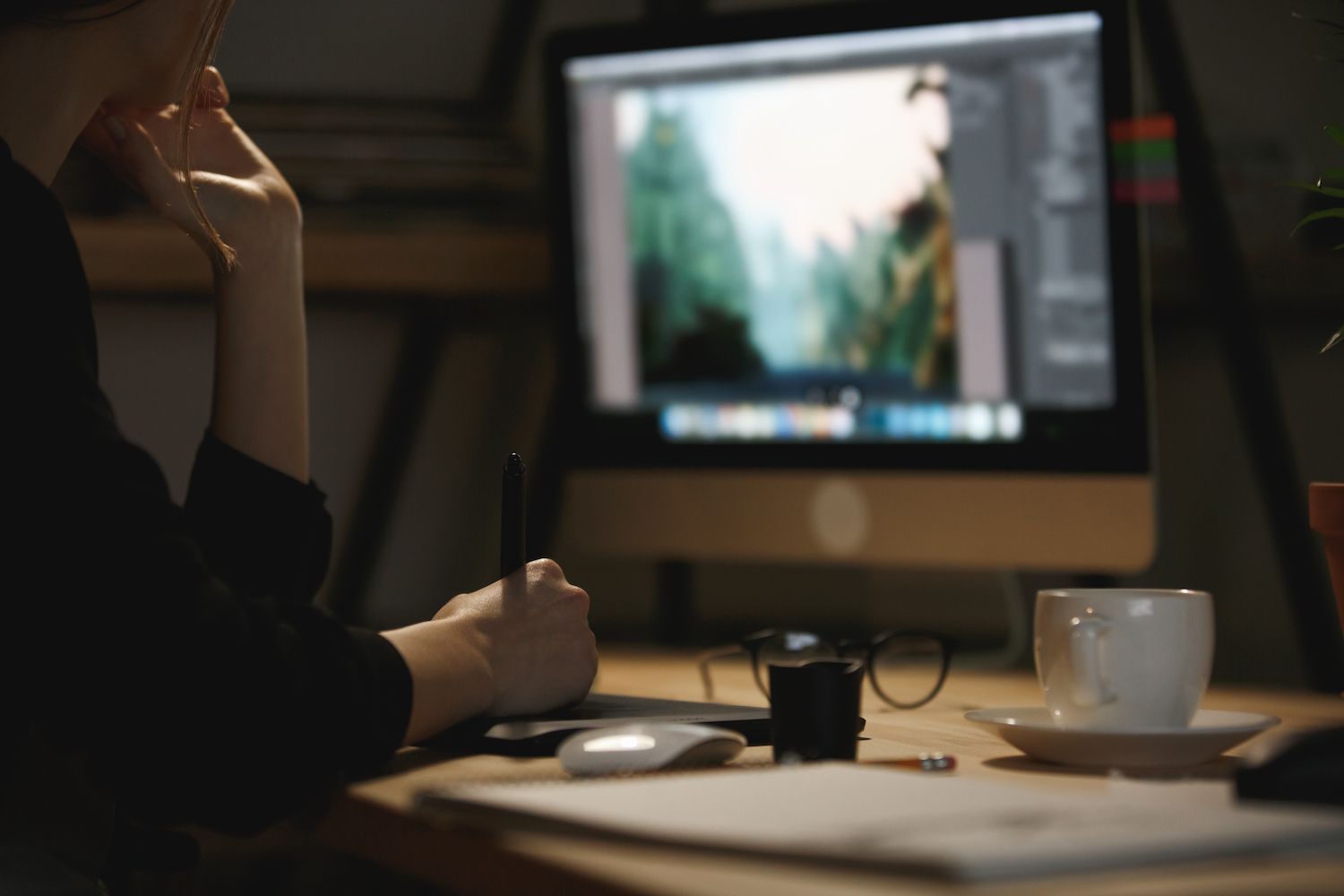
For an React developer, understanding the way React works isn't the only aspect you need to complete for building projects that are easy to use and easy to manage and scale.
Furthermore, you need to be knowledgeable about specific rules and conventions in order to allow you to write short React code. This allows you to aid your clients and assist both you and the developers who work on the project manage the foundation of the code.
In the video tutorial in this guide, we'll begin by discussing some typical challenges React developers face. In the next section, we'll look at various of the most successful ways to code React programs in a better way.
Let's get started!
Challenges React Developers Face
The number of problems in this article can be prevented with a few guidelines which we'll discuss more in detail later.
In this article, we'll begin by talking about one of the most fundamental issues that can befall newbies.
Situations To React
Particularly the fundamental JavaScript concepts and functions that you need to understand prior to starting React. Some of them mostly from ES6 include:
- Arrow is used for a reason.
- Rest operator
- Spread operator
- Modules
- Destructuring
- Methods to array
- Template literals
- Promises
Let
together withconst
variables
The JavaScript areas listed above will provide an understanding of how React functions.
Additionally, you'll learn about the most current concepts inside React like:
- Components
- JSX
- State management
- Props
- Rendering elements
- Event handling
- Conditional rendering
- Lists and keys
- Formulas and validation of Formulas and validation for
- Hooks
- Styling
A thorough understanding of React concepts and the requirements for using the library can allow you to utilize its capabilities efficiently.
State Management
In JavaScript the process of changing a variable may be as simple as assigning it a new value using an operator like ( =
). This is an example
var x = 300; function updateX() x = 100; updateX(); console.log(x); // 100
In the example code you will see that we have made a variable "x"
that has a starting value in the range of 300
.
Employing the equal operator we came up with 100. 100
to it. The code was written with an updateX
function.
In React it's possible to modify the values of variables. This is done in a different manner. Here's how:
load useState from'react'; function App() const [x setX, setX] useState(300) permit updates to =()=> setX(100) then return ( x Update) (x Update X) SetX, setX) SetX, x) SetX setX; export the default App
To update the status of variables in React you can use React's UseState
Hook. There are three things to keep in mind when applying this Hook
- The name of the variable
- This function lets you modify the variable
- The original value or state the variable was at the time of its birth.
In our our example,"x" represents the value assigned for the variable. SetX functions which update the value for x . The contrast is set to (300) for an x. x. This is set as a parameter of the function useState
const [x ], setX, setsX, x[x]. useState(300)
In order to update the status of the x
to reflect the current situation of x, utilized the setX
function:
import useState from'react' Let updateX =()=> (100);
The software updateX
function calls the setX function. setX
function. The function is then employed to set the value of x
to 100.
.
Although this method appears to be an ideal choice for making modifications to the state of variables, it can add to the code that is required to complete large projects. Most State Hooks make the code difficult to trace and understand, particularly for growing projects.
Another issue that comes from this State Hook is that these variables that are created do not get used across the various parts of the application. It's still necessary to have Props to move the information across variables.
For us, it's good to know that there are libraries designed to manage the state of your application in a manner that works together with React. You could even create an individual variable and apply it to any place you'd like inside your React application. The library includes Redux, Recoil, and Zustand.
The problem with using the state management tools of third parties is that you'll need learn concepts which are distinct from the ones you've previously learned with React. Redux provides a great example. It is well-known because of its lengthy boilerplate codes that led to it being difficult for newcomers to understand (although it's been simplified with the Redux Toolkit, which permits users to write less code as opposed to Redux).
Scalability and maintainability
If the demands of the customers alter for the product, there is always a need to modify the software used to create the product.
It's sometimes difficult to expand your code when it's not easy for teams to keep up. These issues arise because of making mistakes when you write the program. The practices might appear to work flawlessly at first giving you the desired result however, what's happening "for the moment" is not efficient to the long-term development of your program.
Responding to the most effective strategies
In this article, we'll go over some of the most effective practices to follow when writing the code to write React code. Let's get started.
1. Make sure that the Folder Structure is clearly defined.
Folder structures help the creators and the end users comprehend the organization of documents and other resources that are used in the design process.
When you've established a solid order for your folders it's simpler to navigate your way to the right location, which will save you time and helping you avoid confusion. The organization of your folders will differ based on the needs of every team. However, these are the most well-known folders that are available in React.
Sorting Folders by Features, or routes
The ability to separate your files by route and capabilities helps you keep everything associated with a specific feature in the same location. In the case, for instance, you have a dashboard that you're using for your users You can store the JavaScript, CSS, and tests files that are related to that dashboard in one folder.
Here's an example to demonstrate that:
dashboard/ index.js dashboard.css dashboard.test.js home/ index.js Home.css HomeAPI.js Home.test.js blog/ index.js Blog.css Blog.test.js
It's evident that one of one of the main features of the application have all their documents and files saved in the same place.
Similar files can be grouped
In addition, you are able to organize similar files within the identical folder. You can also create separate folders in order to store Hooks components Hooks as well as the additional components. Check out this example
hooks/ useFetchData.js usePostData.js components/ Dashboard.js Dashboard.css Home.js Home.css Blog.js Blog.css
There is no need to adhere to these structures when writing the code. If you've come up with a specific arrangement for the files, then use the way you like. So long as the developers have a clear understanding of file format and you're ready to start!
2. Create a Structured Import order
If you're React application continues to grow, it's inevitable that you'll need to create extra imports. Your imports' format can be a big help in aiding you in understanding what is your component.
Common practice is to group the same programs into similar ones. It is generally a good method. It's possible to differentiate the imports of third parties or external sources from imports made locally.
See this illustration:
import Routes, routes imported from "react-router-dom" toimport the createSlice from "@reduxjs/toolkit" and then import the menu from "@headlessui/react" Add the Home folder to "./Home" and import logos and icons from "./logo.svg" Import "./App.css";
In the above example code in the example code above, we have first put the third-party libraries (these were libraries were required to install prior).
We then imported the data we'd created locally, including styles, images as well as components.
For the sake of ensuring easy comprehending and simple use, the example we've picked to present does not have a huge code base but this approach of imports could help other developers understand the layout of your React application better.
It is possible to further organize your local files according to the types of files that you own in the event that you would prefer to organize them that. In other words, it is possible to group images, components styles, hooks, and more, in separate areas within your local imports.
Here is an example:
home is imported to "./Home" and imports "about" within "./About" to import contact from "./Contact" import the logo image file into "./logo.svg" as well as import closeBtn to "./close-btn.svg" in order to load "./App.css" to load "Home.css"
3. Name Conventions. Conventions
Naming conventions can be used to improve the readability of code. The scope of this is not just restricted to components' names as well as the names of your variables, all the way your Hooks.
The React documentation does not provide an official format for name of the components. The most commonly utilized name conventions are found within camelCase along with PascalCase.
PascalCase is used to describe name of component:
import React by importing'react' from function StudentsList() return ( studentList ) export the default studentList
The above component is called StudentList
, which is much easier to read than the StudentList
as well as the Studentlist
.
Do you want to know how we've increased the amount of people who visit our site by 100%?
Join the more than 20,000 subscribers to our weekly newsletter. week. We'll give you insider WordPress techniques!
On the other aspect, this camelCase names convention is primarily employed to distinguish hooks, variables, functions and arrays, as well as functions.
const [firstName, setFirstName] = useState("Ihechikara"); const studentList = []; const studentObject = ; const getStudent = () =>
4. Utilize the Linter
The program analyzes the program you wrote and alerts you the rule that has been in violation. Any word or phrase that is not in compliance with the rule will usually be highlighted red.
Since every developer is unique in their approach to programming, linter tools will help in ensuring that code are consistent.
Tools to Linter will help you to fix bugs swiftly. There are ways to identify spelling mistakes along with variables declared , yet never used as well as additional functions that are similar to these. Certain bugs could be solved in a hurry by changing the software.
5. Employ Snippet Libraries
Snippet libraries aid developers in developing faster by offering prebuilt codes that developers are able to use regularly.
A good example is the ES7+ React/Redux/React-Native snippets extension, which has a lot of helpful commands for generating prebuilt code. In the instance of, for instance, wanting to create an React functional component, and not having to write the entire code All you have to input is "rfce"
and then press. Enter.
The command above will go further to create a functioning component with an alias which matches the file's title. We generated the code below using the ES7+ React/Redux/React-Native snippets extension:
import React using the call to'react studentList method() return ( studentList ) export default StudentList
Another option for small snippets code that can be useful is an extension called the Tailwind CSS IntelliSense extension, that aids in the creation of web pages that are styled with Tailwind CSS. This extension aids you in autocompletion, providing useful classes for example, syntax highlight or functions to lint. This extension will allow you to look at the color scheme when you type.
6. Combining CSS together with JavaScript JavaScript
In the case of large projects when working on large projects, the use of separate styles of stylesheet files to separate components can create a mess in the way of organizing files difficult to be navigated by users.
A solution to this is mixing the CSS and JSX code. You can use frameworks libraries or frameworks like Tailwind CSS and Emotion for this.
This is how the styling that is created by Tailwind CSS appears:
edge of resource
The following code gives paragraph elements in bold type and gives some space to the right. This is possible this thanks to the utility classes in the framework.
Here's how you'd design an element with Emotion:
Hello World!
7. Limit Component Creation
One of the main characteristics of React is the code reusability. You can build your own components and then use the logic for the number of times you'd like, without having to change the algorithm.
Be aware of this when making your files, it's essential to reduce the amount of parts you create. Doing so will create a massive structure for your files that contain redundant types of files that do not need to be present at all.
Let's take a very simple diagram to show this:
Function UserInfo() return ( My name is Ihechikara. ) • Export the default UserInfo
This image displays the user's name. If we were able to create only one file per user, this would create excessive amount of documents. (Of course , having user data is utilized makes the process simpler. In real-world situations there may be a need to employ a different way of thought.)
In order to make our work usable, we could use props. Here's how:
Function UserInfo(UserName) *Export default UserInfo
Once we have that done and we're done, we'll be able to use this part and import it for the number of times we'd like.
move UserInfo into "./UserInfo" Function Application() return ( ) to export the default app
Three distinct instances that illustrate three distinct examples of UsersInfo
component that derives from the source code stored in a single file instead of having separate files for each user.
8. Implement Lazy Loading
In default, React bundles and then deploys the entire application. You can change this behaviour through lazy loading or code split.
It is possible to restrict the contents of your app to be loaded by an exact date. This can be done through breaking the bundles and loading the information that meets the needs of users. For instance, you may start by loading just the required process to allow users to register, and then transfer the required logic onto the dashboard when logins have been successfully completed by users to the system.
9. Utilize Reusable Hooks
Hooks built into React allow you to harness the extra capabilities of React such as interfacing with the state of your component and running effects in relation to changes in the state of the component. This can be done without the need the ability to create classes for your components.
Additionally, we can make Hooks reusable so that they don't require writers to create the code each time they are used in. This is to create distinct Hooks that are able to be added at any time in the application.
As you can see In the next instance we'll make a Hook to fetching information from APIs which aren't internal
utilize useState as well as useEffect using "react";function useFetchData(url) Const [data setDatadata ] SetData useState(null) setData useState, setData useState (null )(() = useEffect (()) =fetch(url) .then((res) (res) = res.json()) .then((data) is setData(data)) .catch((err) > console.log(`Error in the $err `)); [url]) Export data; return the default value for useFetchData.
The Hook was created that can get data from the APIs mentioned in the previous paragraphs. The hook can be integrated to any part that is an element of your system. This means that you don't have to create all the process within each component that demands external information.
The number of custom Hooks which you're able to create in React is endless, therefore you are able to choose the most effective method to utilize them. Be aware that when creating features that have been designed to work across different components, it is important to ensure that the feature is reuseable.
10. Track and Control Errors
There are different ways of handling errors in React like using error boundaries, try and catch blocks or using external libraries like react-error-boundary
.
The built-in error boundary that were implemented within React 16 was a functionality intended specifically for classes, so we won't discuss it since it's recommended to utilize functional components instead of class components.
But the attempt
and catch
block is for to execute imperative code but it's not a declaration process. It's therefore not a viable option with JSX.
Our best recommendation would be to use a library like react-error-boundary. The library offers functions wrapped around the component you are using, and will help you identify problems while the React application is rendered.
11. Check and confirm your Code
Although many would argue that testing shouldn't be a concern when developing an app for the internet, there are many benefits to doing it. Here are a few examples:
- The discovery of bugs leads to improved code quality.
- Unit tests can be documented in order to gather data for future sources.
- The simple solution to bugs may help users avoid having the expense of paying developers to remove the fire which the flaw can cause if the issue isn't addressed.
12. Utilize Functional Components
Functional components using functional components React offers a variety advantages: There's less writing required and it's easy to comprehend and the beta version of official React document. The document is being improved by using functional components (Hooks) which is why it's a good idea to get used to working using functional components.
Functional components will ensure that there is no need to think about the best way to use the
or use classes. You can also keep track of the state of your program by writing much less code using Hooks.
A majority of the information that's updated available through React uses functional elements. This makes it easy to comprehend and follow the helpful guides and other resources produced by members of the community when there are problems.
13. Be Up-to-Date with Updates to React Version
Over the next few times, new features will be introduced, and old ones will be modified. One way to keep track of this is to keep an eye on the official documentation.
Additionally, you can join React community groups on social media to receive updates on developments as they occur.
Keep up-to-date to the most recent version of React can allow you to determine the best times you should optimize or modify the base code to ensure most efficacious performance.
In addition, there are additional libraries built on React which you must remain current with and utilize, such as React Router, which is utilized to move data through React. Being aware of the changes the libraries make could aid you in making the necessary changes to your program and make the process easier for everybody working on the project.
Furthermore, certain functions may be removed, as well as some terms might change as new versions become more widely available. To stay on the safer side, you should always go through the user instructions and the manuals to check for any changes.
14. Make use of a fast and safe Hosting Service
If you'd like to make your site application accessible to everyone after you've created it, you'll need to host it. You must select the most reliable and secure hosting service.
Hosting your site gives access to a range tools to help you control and expand your site easily. The website server that hosts your website is hosted permits all data stored is stored locally on your computer to be safely stored within the server. The main benefit having your site hosted is that visitors can view the awesome stuff that you've put together.
There's an array of web hosting companies that offer free hosting for developers, like Firebase, Vercel, Netlify, GitHub Pages, or additional services that are more expensive like Azure, AWS, GoDaddy, Bluehost, and so on.
Summary
While you're developing your next app for the web using React follow these tips with you to ensure that controlling and using the application is effortless for both users and your development team.
What other React best practices are there that you aren't aware of however weren't covered in this piece? Comment in the comments below. Have fun coding!
- It's easy to set up and manage My dashboard. My dashboard
- Help is always on hand.
- The top Google Cloud Platform hardware and network is driven by Kubernetes to provide the highest capacity
- Top-of-the-line Cloudflare integration that improves speed as well as security
- The global reach is facilitated by more than 35 data centers, plus 275 POPs across the world
The article was first seen here
Article was posted on here