What can you do to create an app to detect living objects with React? (r) (r)
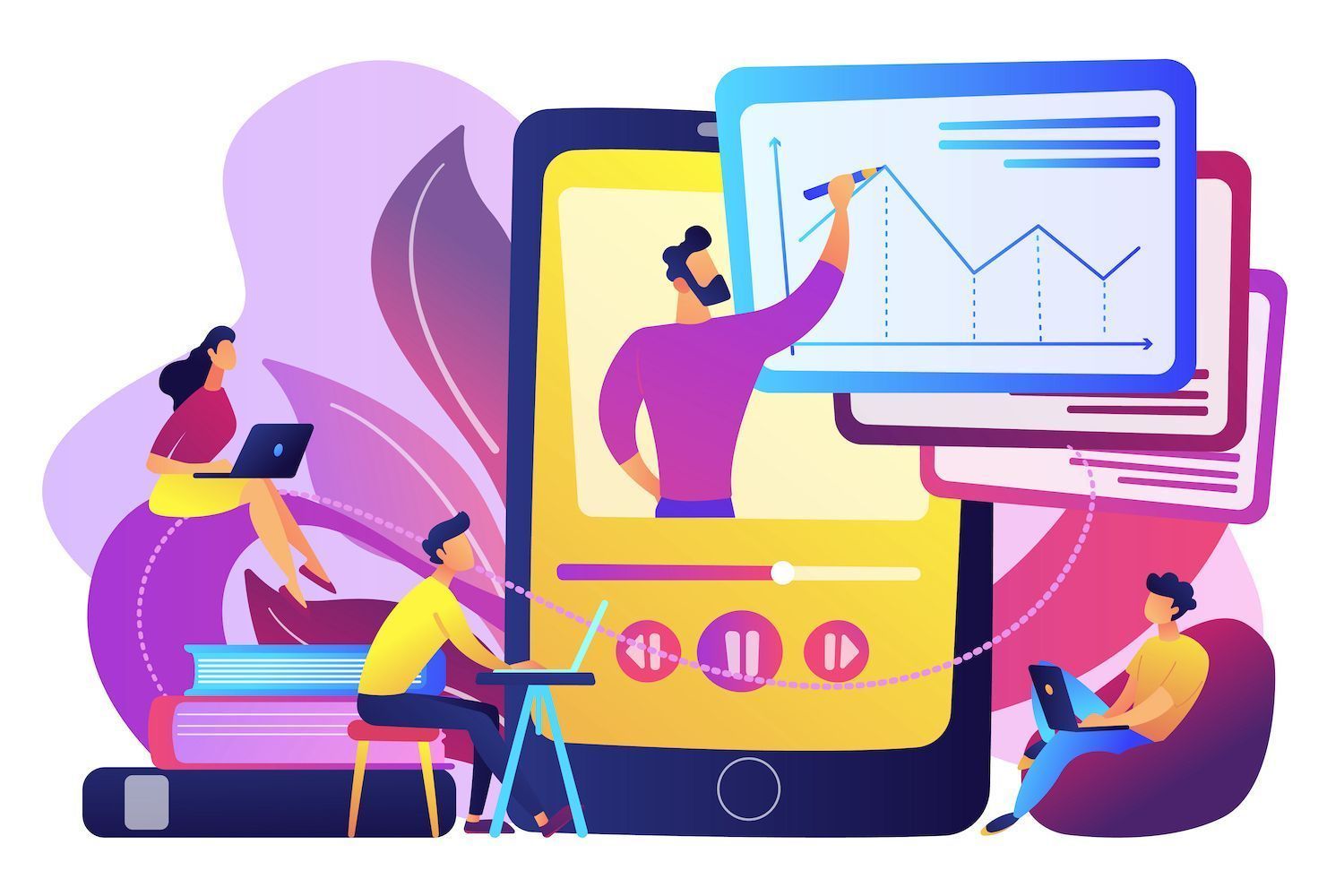
-sidebar-toc>
Cameras are becoming more sophisticated and becoming more sophisticated, real-time object detection is becoming a well-known option. From autonomous vehicles, to intelligent surveillance systems, to AR applications, this technology is utilized in a variety of applications.
Computer vision is a vague word used to describe the process that makes use of cameras and computers for the various operations. previously mentioned, is a huge and complicated area. A lot of people don't realize that it's possible to begin participating in the instant search for objects by using your web browser.
The situation
This is a list of the most important technologies used for this article:
- TensorFlow.js: TensorFlow.js is a JavaScript library that can bring the benefits of machine learning to your browser. It lets you download already developed models which have been trained to perform object recognition and then run them in the browser. It removes the need to perform complex server-side processing.
- Coco SSD The application utilizes an object recognition model that has been trained. It is known is known as Coco SSD that is a lightweight model that is capable of recognising the vast majority of objects that are commonplace instantly. Although Coco SSD is a powerful instrument, you should be aware that it is created using a variety of different objects. If you've got particular need for detection, then you may develop a customized model by using TensorFlow.js by the following tutorial.
Start a new React project
- Make a brand fresh React project. It is possible to do this with these instructions:
NPM create vite@latest -object detection React template
This will generate an initial React project that you can create with it..
- Then, you'll be able to Install TensorFlow and The Coco SSD libraries by running these commands in the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
It is now the right best time to develop your application.
Configuring the app
Prior to writing the code to create the logic needed to recognize objects, take a take a look at the code was created in this tutorial. The user interface for the app might be:
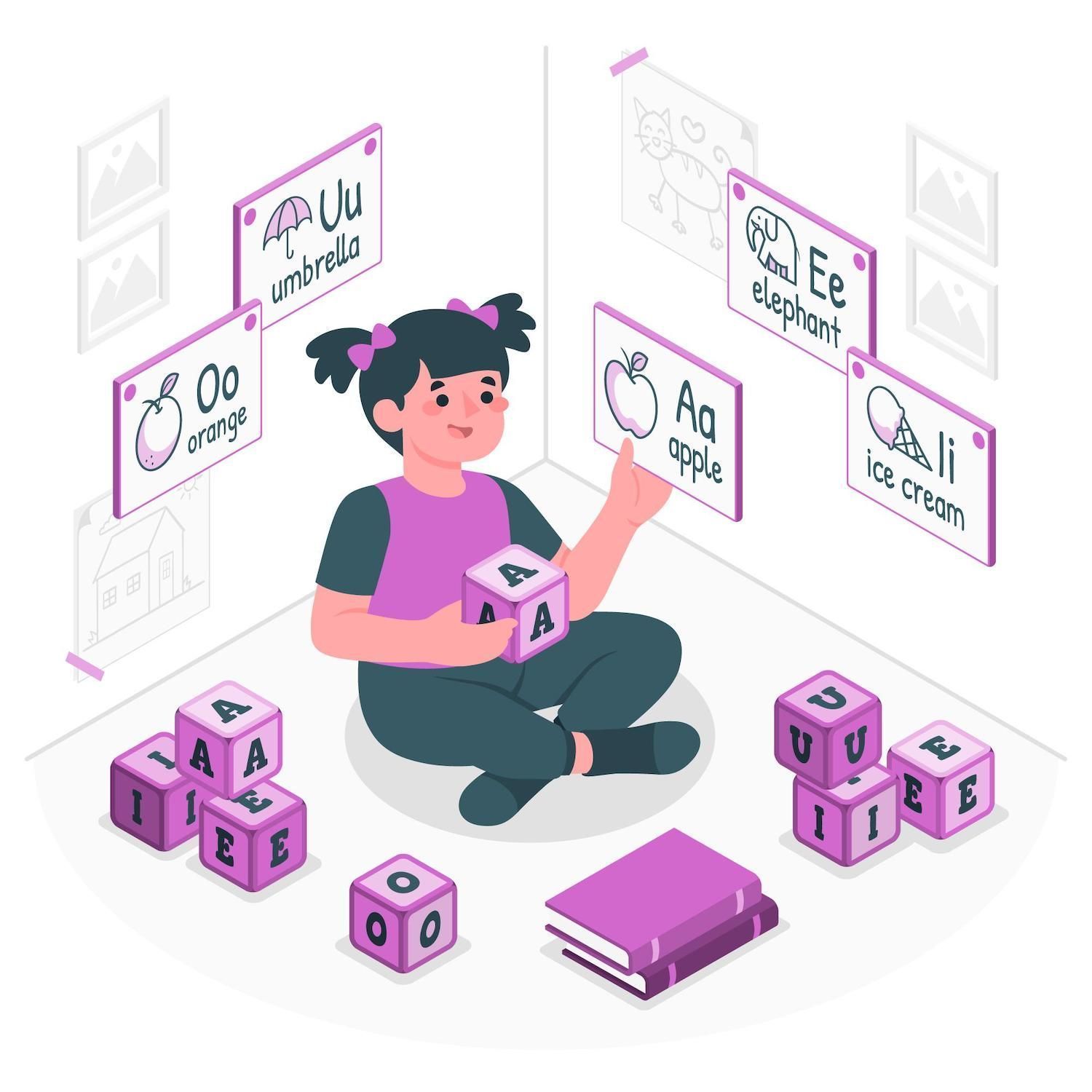
Users press the Start Webcam button when they click the Start Webcam button, they're requested to authorize the app to use webcam feeds. Once permission has been granted, the application will begin show the live stream from the webcam. It will also recognize the items it sees in the feed. Following that, it constructs an equilateral triangle to show what it sees in the feed, and labels them. Then, then, it labels them.
The first step is to create a user-friendly user interface for your application. Then, copy these steps into app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
The code fragment is the header for the page. The code also incorporates a customised component called "ObjectDetection"
. It will take the feed of a webcam, and then locating objects on a moment's notice.
In order to create this component create a brand-new document named ObjectDetection.jsx in your homedirectory and copy the following code in it:
UseEffect as well as useState from'react'. Const objectDetection = () //const videoRef = useRef(null) Const [isWebcamStarted], setIsWebcamStarted] = useState(false) Const setWebcam to be async () = /StopWebcam = () > // TODO; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
This is how you can use the code to make startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system will request users to grant access rights to their webcam and once granted, the system will change video. video
is used to display the live feed from the webcam to anyone accessing the service.
If the program is unable to connect to the camera feed (possibly because of the lack of a webcam installed in the device or another reason for why the user was not granted access) it will display an error message to the console. The console could print an error message that will explain the reason for the issue for the user.
The next step is to substitute the stopWebcam
function using this code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code searches for videos that are accessible via the video
object and stops every one of them. Then, it changes to change the isWebcamStarted
condition in the status to true
.
If you are in this situation it is possible to run the application to see if it is able to access and see the feed on the webcam.
You must paste the code within the index.css file to ensure that your application looks exactly as the one you saw earlier
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
You must delete the App.css file to ensure you don't ruin the look of your elements. You are now ready to implement the necessary technology of real-time object recognition into your app.
Install real-time detection of objects
- Initial steps are to import the data from Tensorflow along with Coco SSD at the top of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Create a new condition in the
ObjectDetection
component, in order to preserve the prediction array that is generated by the Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- You can then develop a program that loads into the Coco SSD model. It then loads into Coco SSD model, loads onto Coco SSD model, collects footage, and predicts:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
This program makes use of the feed of video to create predictions about objects that are in the feed. The program gives you an assortment of objects predicted to be visible, each featuring a tag that includes a percentage of the confidence level and numbers that indicate the location of the object inside the video's frame.
It is essential to invoke this feature to process videos as frames are added and afterwards use the forecasts that are stored in the forecasts
state to show boxes and labels for every known object in the video stream that is currently live.
- After that, you'll be in a position to utilize
setInterval
function to call this function on a regular schedule. Also, you must make sure that this function is not active after the user is shut off from feeds from their webcam. To stop the possibility of this happening, you must utilize yourClearInterval
functionality within JavaScript.Add the state container and useEffect hooks to theeffect
hooks in theelement the object detection
element, to develop thepredictObject
function. This program runs indefinitely when the webcam's on but is then taken off of the webcam after it is shut off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
The app is set up to identify objects in the image of the camera each 500 milliseconds. It's possible to change the milliseconds per second based on how fast you'd like the object detect speed however, be mindful of the possibility of using it too frequently. could result in your program taking up a substantial amount of memory within the browser.
- When you've got the data for your prediction then you're in a position to use the information in order to create prediction. If you have a forecast, it can be used to state containers. You can use it to show the label, and even use it to be used as a container on the live feed in your film. To do this, it's necessary to modify the
return
declaration for yourlabel detection.
Enter the following information:
Return ( isWebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program displays the forecast list underneath the webcam's feed. The program then creates an space around the object forecasted which includes coordinates for Coco SSD as well as names at the bottom of each box.
- For styling the boxes and labels properly For styling the labels and boxes correctly, add this code to index.css Index.css file: index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is complete. It is complete. The program has been able to start the server that developed it to run tests on the program. What happens when the program has been completed
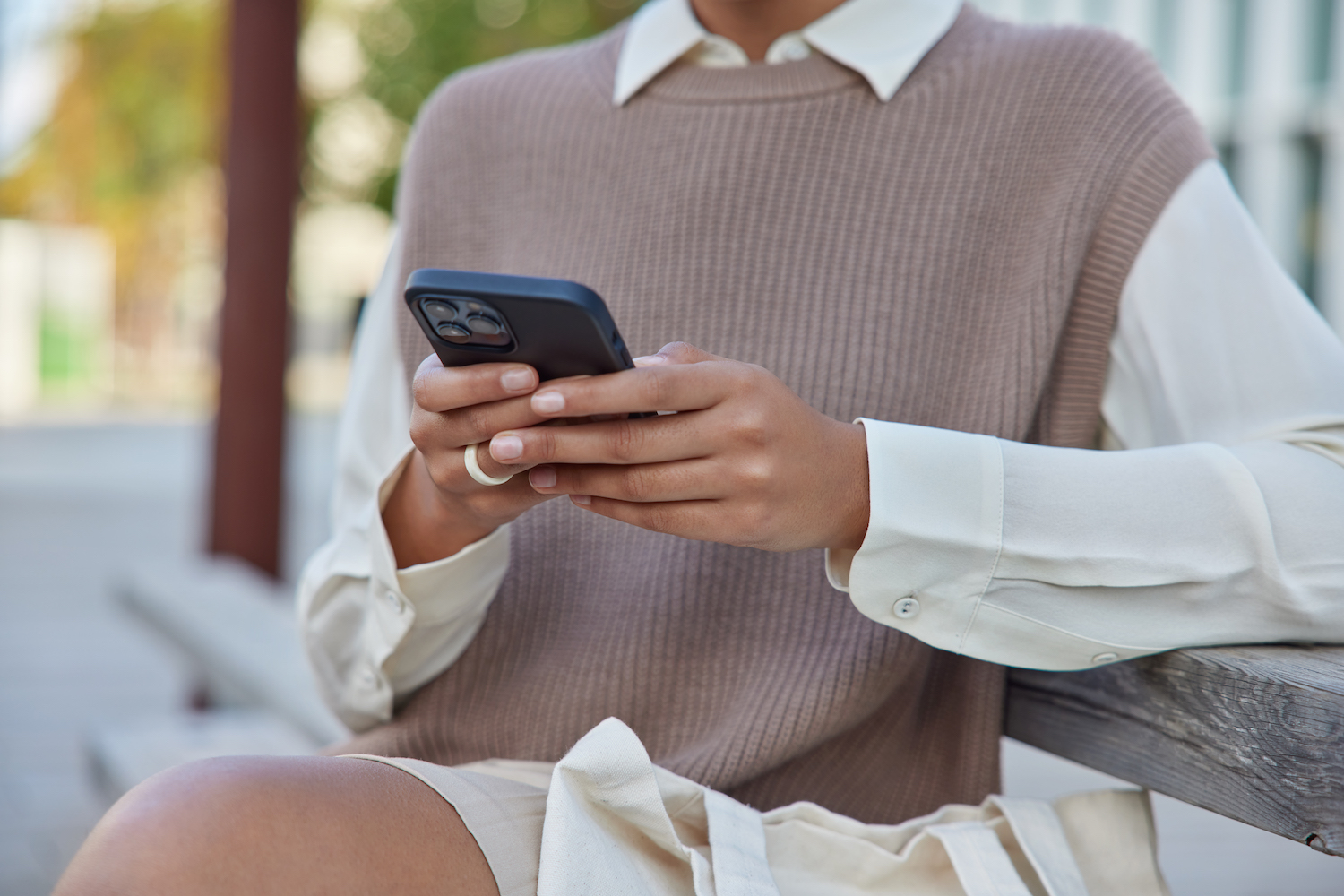
Complete code is available is available in the repository on GitHub. GitHub repository.
Install the app
If your repository on Git is up and running, proceed to these steps for installing the application :
- Sign in or create an account in order to view the dashboard on Your Dashboard. My dashboard.
- Authorize with your Git provider.
- Choose those static websites in the sidebar to the left and choose Add Site. Select Add Site.
- Choose the branch and the repository you want to be able to access through.
- Assign a unique name to your site.
- Integrate the setting for building according to the following format:
- Command to build:
yarn build
orNPM build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
After the app has been launched After the app is launched, you can click "Visit the website" from the dashboard to launch the application. The app can be tested on different cameras of various devices to see how it performs.
Summary
It's been very successful in developing the object detection technology in real time as well as live-time application by made with React, TensorFlow.js, and . This allows you to investigate the potential of computer vision and create interactive experiences in your browser.
Take note that the Coco SSD model we used can only be a base. If you'd like to continue exploring the possibilities look into the possibility of being able to alter the method of detecting objects using TensorFlow.js that allows you to customize the app to identify the most appropriate objects that meet the specific needs of your business.
There are no limits to what you can do! The app can be the foundation for creating advanced applications like Augmented Reality Experiences, as well as advanced surveillance techniques. If you can launch your app via the secure platform, it is possible to communicate your creation with anyone around the globe, and watch the potentialities of computer vision come into the forefront.
What's the most difficult issue you've encountered which you think the real-time detection of objects can help solve? Write about your experiences in the comment section below!
Kumar Harsh
Kumar is an author of technical software and also a journalist with his location in India. He's an expert on JavaScript and DevOps. Get more information about his experience through his site.
The article originally appeared on on this web site.
Article was posted on here
This post was posted on here